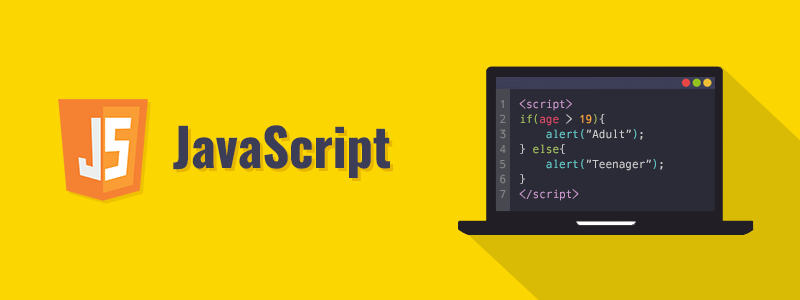
Regular expressions (RegExp) are powerful tools used in JavaScript for pattern matching and text manipulation. They help developers search, validate, and manipulate strings efficiently. This guide will cover everything you need to know about JavaScript Regular Expressions, from creating them to using common patterns and methods.
1. Creating Regular Expressions
In JavaScript, there are two ways to create a regular expression:
Using a Regular Expression Literal
let regex = /pattern/flags;
- Example:
let pattern = /hello/i; // 'i' flag makes the search case-insensitive
Using the RegExp Constructor
let regex = new RegExp("pattern", "flags");
- Example:
let pattern = new RegExp("hello", "i");
2. Common Patterns
Regular expressions use special characters and sequences to define search patterns. Here are some commonly used ones:
Character Classes
.
– Matches any character except a newline.\d
– Matches any digit (0-9).\D
– Matches any non-digit character.\w
– Matches any word character (a-z, A-Z, 0-9, _).\W
– Matches any non-word character.\s
– Matches whitespace (space, tab, newline).\S
– Matches any non-whitespace character.
Anchors
^
– Matches the start of a string.$
– Matches the end of a string.
Quantifiers
*
– Matches 0 or more occurrences.+
– Matches 1 or more occurrences.?
– Matches 0 or 1 occurrence.{n}
– Matches exactlyn
occurrences.{n,}
– Matchesn
or more occurrences.{n,m}
– Matches betweenn
andm
occurrences.
Groups and Ranges
(abc)
– Matches exactly "abc".[a-z]
– Matches any lowercase letter.[A-Z]
– Matches any uppercase letter.[0-9]
– Matches any digit.(x|y)
– Matches either "x" or "y".
Escaping Special Characters
If you need to match a character that has a special meaning in RegExp, you must escape it using \
. For example, to match a literal .
(dot), use \.
.
3. Methods for Working with Regular Expressions
JavaScript provides several built-in methods for working with regular expressions.
1. test()
The test()
method checks if a pattern exists in a string. It returns true
or false
.
let regex = /hello/i; console.log(regex.test("Hello, world!")); // true
2. match()
The match()
method returns an array containing the matches found in a string.
let text = "JavaScript is amazing!"; let pattern = /is/; console.log(text.match(pattern)); // ["is", index: 11, input: "JavaScript is amazing!", groups: undefined]
3. replace()
The replace()
method replaces matched text with a specified replacement.
let text = "I love JavaScript!"; let pattern = /JavaScript/; console.log(text.replace(pattern, "Python")); // "I love Python!"
Using global flag g
to replace all occurrences:
let text = "Apple, apple, APPLE!"; let pattern = /apple/gi; console.log(text.replace(pattern, "banana")); // "banana, banana, banana!"
4. exec()
The exec()
method searches for a match in a string and returns an array or null
if no match is found.
let regex = /hello/i; let result = regex.exec("Hello, world!"); console.log(result); // ["Hello", index: 0, input: "Hello, world!", groups: undefined]
Conclusion
JavaScript Regular Expressions are essential for text processing, validation, and pattern matching. By mastering how to create RegExp, use common patterns, and apply methods like test()
, match()
, replace()
, and exec()
, you can efficiently handle string manipulations in JavaScript.
Start practising with RegExp in your projects, and soon you'll be using them like a pro!
Leave a Comment