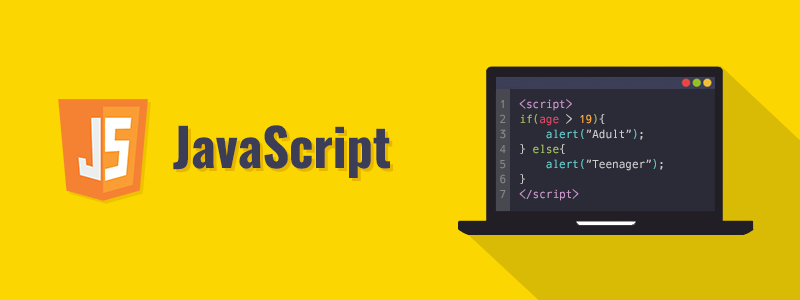
JavaScript is a dynamic and versatile language used for both frontend and backend development. To write maintainable, scalable, and efficient code, developers often leverage design patterns. Design patterns are reusable solutions to common programming problems. In this blog, we will explore four key JavaScript design patterns:
- Module Pattern
- Factory Pattern
- Singleton Pattern
- Observer Pattern
Let's dive into each pattern in detail with explanations and code examples.
1. Module Pattern
The Module Pattern helps in organizing code by encapsulating related functions, variables, and methods within a single unit. It prevents polluting the global scope and promotes reusability and maintainability.
Example:
const CalculatorModule = (function() { let result = 0; return { add: function(num) { result += num; return result; }, subtract: function(num) { result -= num; return result; }, getResult: function() { return result; } }; })(); console.log(CalculatorModule.add(5)); // Output: 5 console.log(CalculatorModule.subtract(2)); // Output: 3 console.log(CalculatorModule.getResult()); // Output: 3
Benefits:
- Encapsulates variables and methods.
- Prevents namespace pollution.
- Provides a clear structure for organizing code.
2. Factory Pattern
The Factory Pattern is a creational design pattern that provides a way to create objects without specifying the exact class of the object. It is useful when dealing with object creation complexity.
Example:
function CarFactory(type) { const car = {}; car.type = type; if (type === "SUV") { car.wheels = 4; car.drive = function() { return "Driving an SUV"; }; } else if (type === "Truck") { car.wheels = 6; car.drive = function() { return "Driving a Truck"; }; } return car; } const suv = CarFactory("SUV"); console.log(suv.drive()); // Output: Driving an SUV const truck = CarFactory("Truck"); console.log(truck.drive()); // Output: Driving a Truck
Benefits:
- Simplifies object creation.
- Reduces code duplication.
- Decouples object creation logic from the main program.
3. Singleton Pattern
The Singleton Pattern ensures that only one instance of a class exists at any given time. This pattern is useful for managing shared resources such as database connections or configuration settings.
Example:
const Singleton = (function() { let instance; function createInstance() { return { message: "I am the single instance!" }; } return { getInstance: function() { if (!instance) { instance = createInstance(); } return instance; } }; })(); const instance1 = Singleton.getInstance(); const instance2 = Singleton.getInstance(); console.log(instance1 === instance2); // Output: true console.log(instance1.message); // Output: I am the single instance!
Benefits:
- Ensures a single point of access.
- Saves memory by reusing an existing instance.
- Useful for managing shared resources.
4. Observer Pattern
The Observer Pattern is a behavioural pattern where an object (subject) maintains a list of dependents (observers) and notifies them of any state changes. It is commonly used in event handling and reactive programming.
Example:
class Subject { constructor() { this.observers = []; } subscribe(observer) { this.observers.push(observer); } unsubscribe(observer) { this.observers = this.observers.filter(obs => obs !== observer); } notify(data) { this.observers.forEach(observer => observer.update(data)); } } class Observer { constructor(name) { this.name = name; } update(data) { console.log(`${this.name} received update: ${data}`); } } const subject = new Subject(); const observer1 = new Observer("Observer 1"); const observer2 = new Observer("Observer 2"); subject.subscribe(observer1); subject.subscribe(observer2); subject.notify("New data available!"); // Output: // Observer 1 received update: New data available! // Observer 2 received update: New data available!
Benefits:
- Enables loose coupling between objects.
- Allows multiple observers to react to changes in the subject.
- Commonly used in event-driven applications.
Conclusion
Understanding and implementing design patterns can significantly improve the structure and efficiency of JavaScript applications. Here’s a quick recap of what we covered:
- Module Pattern: Encapsulates related code into a single unit.
- Factory Pattern: Creates objects without specifying their exact class.
- Singleton Pattern: Ensures only one instance of an object exists.
- Observer Pattern: Enables objects to subscribe and react to changes.
By mastering these patterns, developers can write cleaner, modular, and maintainable JavaScript code. Happy coding!
Leave a Comment