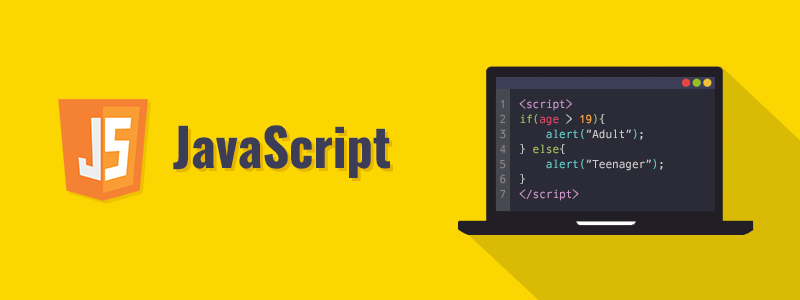
Introduction
Web storage is an essential part of modern web development, allowing developers to store data in the user's browser efficiently. JavaScript provides three primary methods for web storage:
- LocalStorage ā Stores data with no expiration time.
- SessionStorage ā Stores data for the duration of a session.
- Cookies ā Stores small amounts of data that can be sent with HTTP requests.
In this blog, we'll explore how each method works, their advantages, disadvantages, and when to use them.
1. LocalStorage
What is LocalStorage?
LocalStorage is a storage mechanism that allows data to be stored in a user's browser with no expiration. The data persists even after the browser is closed and reopened.
Key Features:
- Stores key-value pairs.
- Persistent storage (data remains unless explicitly removed).
- Maximum storage capacity is around 5MB.
- Cannot be accessed from different domains (same-origin policy applies).
How to Use LocalStorage
Setting Data:
localStorage.setItem("username", "JohnDoe");
Retrieving Data:
let user = localStorage.getItem("username"); console.log(user); // Output: JohnDoe
Removing Data:
localStorage.removeItem("username");
Clearing All Data:
localStorage.clear();
When to Use LocalStorage:
- Storing user preferences.
- Caching small amounts of data.
- Saving application settings.
Disadvantages:
- Cannot store complex objects (must be serialized into strings using
JSON.stringify
). - Can be accessed by JavaScript, making it vulnerable to XSS (Cross-Site Scripting) attacks.
2. SessionStorage
What is SessionStorage?
SessionStorage is similar to LocalStorage but only stores data for the duration of a session. Data is lost when the browser tab is closed.
Key Features:
- Stores key-value pairs.
- Data persists only until the session ends (browser tab is closed).
- Maximum storage capacity is around 5MB.
How to Use SessionStorage
Setting Data:
sessionStorage.setItem("sessionUser", "JaneDoe");
Retrieving Data:
let sessionUser = sessionStorage.getItem("sessionUser"); console.log(sessionUser); // Output: JaneDoe
Removing Data:
sessionStorage.removeItem("sessionUser");
Clearing All Data:
sessionStorage.clear();
When to Use SessionStorage:
- Storing temporary data that should be removed once the user leaves the site.
- Handling multi-page form submissions.
Disadvantages:
- Data is lost when the browser tab is closed.
- Cannot be accessed from different domains.
3. Cookies
What are Cookies?
Cookies are small text files stored in the browser and sent with HTTP requests. They are mainly used for session management, tracking, and authentication.
Key Features:
- Stores up to 4KB of data.
- Can have an expiration date.
- Can be accessed by the server and client.
- Sent with every HTTP request, which can affect performance.
How to Use Cookies
Setting a Cookie:
document.cookie = "user=JohnDoe; expires=Fri, 31 Dec 2025 12:00:00 UTC; path=/";
Retrieving a Cookie:
console.log(document.cookie);
Deleting a Cookie:
document.cookie = "user=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/";
When to Use Cookies:
- Authentication (e.g., storing session tokens).
- User tracking and analytics.
- Remembering user preferences across visits.
Disadvantages:
- Limited storage capacity (4KB).
- Sent with every HTTP request, which can impact performance.
- Can be accessed by JavaScript, making them vulnerable to XSS attacks.
Comparison Table
Conclusion
Each web storage method has its use cases:
- Use LocalStorage for persistent client-side data storage.
- Use SessionStorage for temporary session-based data.
- Use Cookies for storing small pieces of data that need to be sent with HTTP requests, such as authentication tokens.
Understanding these differences helps developers choose the best storage solution based on their application's needs. By leveraging web storage effectively, you can enhance performance, security, and user experience.
Do you use web storage in your projects? Share your experiences and thoughts in the comments below!
Leave a Comment