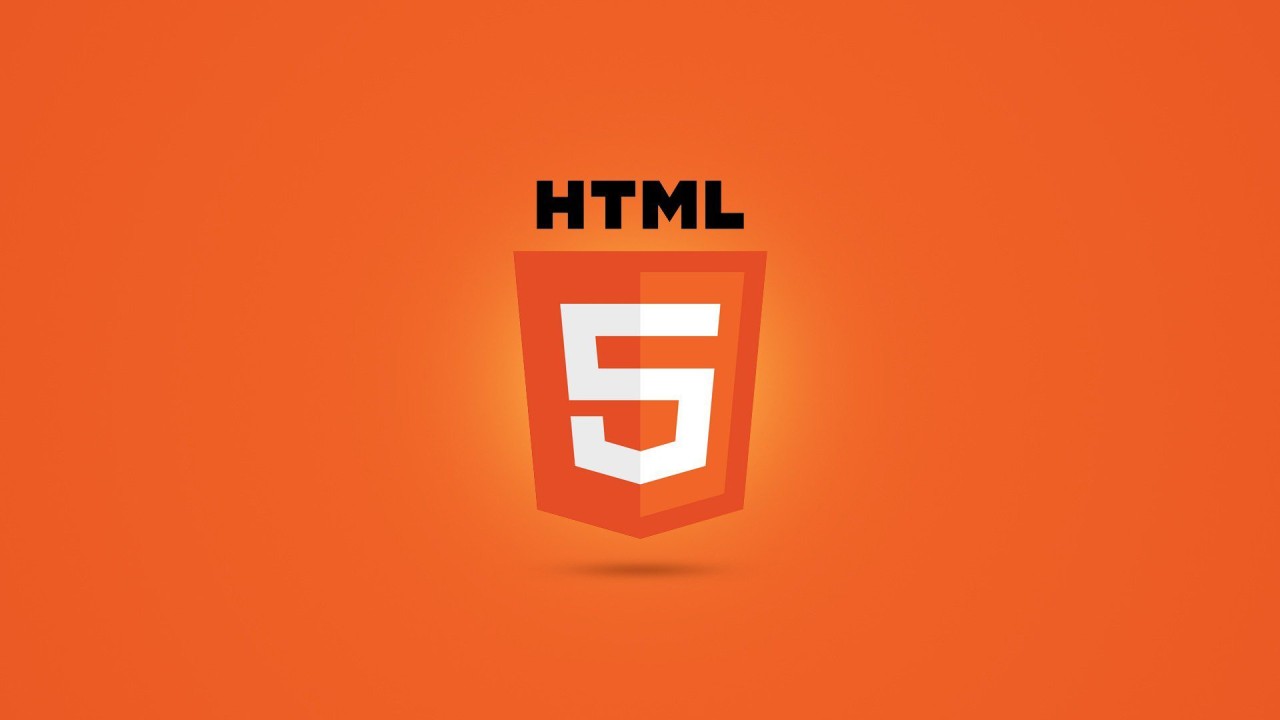
HTML5 introduced several powerful APIs that enhance web applications' functionality and user experience. In this blog, we will explore five essential HTML APIs: Geolocation API, Drag and Drop API, Web Storage API (LocalStorage & SessionStorage), WebSockets API (Basic Overview), and Fetch API (Basic Overview).
1. Geolocation API
The Geolocation API allows web applications to access the user's geographic location. It is commonly used for location-based services, such as maps, navigation, and personalized content.
Example Usage:
if (navigator.geolocation) { navigator.geolocation.getCurrentPosition( (position) => { console.log(`Latitude: ${position.coords.latitude}, Longitude: ${position.coords.longitude}`); }, (error) => { console.error("Error getting location: ", error.message); } ); } else { console.log("Geolocation is not supported by this browser."); }
Key Features:
- Supports real-time location tracking.
- Works with both desktop and mobile browsers.
- Requires user permission for privacy.
2. Drag and Drop API
The Drag and Drop API allows users to click and drag elements within a web page. It is commonly used in file uploads, task management applications, and interactive UIs.
Example Usage:
<div id="dragItem" draggable="true">Drag Me</div> <div id="dropZone">Drop Here</div> <script> const dragItem = document.getElementById("dragItem"); const dropZone = document.getElementById("dropZone"); dragItem.addEventListener("dragstart", (event) => { event.dataTransfer.setData("text", event.target.id); }); dropZone.addEventListener("dragover", (event) => { event.preventDefault(); }); dropZone.addEventListener("drop", (event) => { event.preventDefault(); const data = event.dataTransfer.getData("text"); dropZone.appendChild(document.getElementById(data)); }); </script>
Key Features:
- Enables smooth drag-and-drop interactions.
- Supports transferring files and elements.
- Requires event handling for
dragstart
,dragover
, anddrop
.
3. Web Storage API (LocalStorage & SessionStorage)
The Web Storage API provides a way to store key-value pairs in the browser. It consists of:
- LocalStorage: Stores data with no expiration (persists across sessions).
- SessionStorage: Stores data for a single session (cleared when the tab is closed).
Example Usage:
// Store data in LocalStorage localStorage.setItem("username", "JohnDoe"); console.log(localStorage.getItem("username")); // Outputs: JohnDoe // Store data in SessionStorage sessionStorage.setItem("sessionID", "ABC123"); console.log(sessionStorage.getItem("sessionID")); // Outputs: ABC123
Key Features:
- Stores data in key-value format.
- LocalStorage data persists even after closing the browser.
- SessionStorage data is removed when the session ends.
4. WebSockets API (Basic Overview)
The WebSockets API enables real-time communication between a client and a server using a persistent connection. Unlike HTTP requests, WebSockets allow bidirectional data transfer without reloading the page.
Example Usage:
const socket = new WebSocket("wss://echo.websocket.org"); socket.onopen = () => { console.log("WebSocket connection established"); socket.send("Hello, WebSocket!"); }; socket.onmessage = (event) => { console.log("Received: ", event.data); }; socket.onclose = () => { console.log("WebSocket connection closed"); };
Key Features:
- Enables real-time chat, live notifications, and multiplayer gaming.
- Reduces network overhead compared to repeated HTTP requests.
- Supports full-duplex communication.
5. Fetch API (Basic Overview)
The Fetch API provides a modern way to make HTTP requests asynchronously. It replaces the older XMLHttpRequest
method and is widely used for interacting with RESTful APIs.
Example Usage:
fetch("https://jsonplaceholder.typicode.com/posts/1") .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error("Error fetching data:", error));
Key Features:
- Supports promise-based syntax.
- Handles GET, POST, PUT, DELETE requests.
- Allows custom headers and error handling.
Conclusion
HTML5 APIs provide powerful features that enhance web applications, offering better user experiences and improved performance. The Geolocation API enables location-based services, the Drag and Drop API improves UI interactions, the Web Storage API allows efficient data storage, the WebSockets API supports real-time communication, and the Fetch API simplifies HTTP requests. By leveraging these APIs, developers can build dynamic and efficient web applications.
Leave a Comment