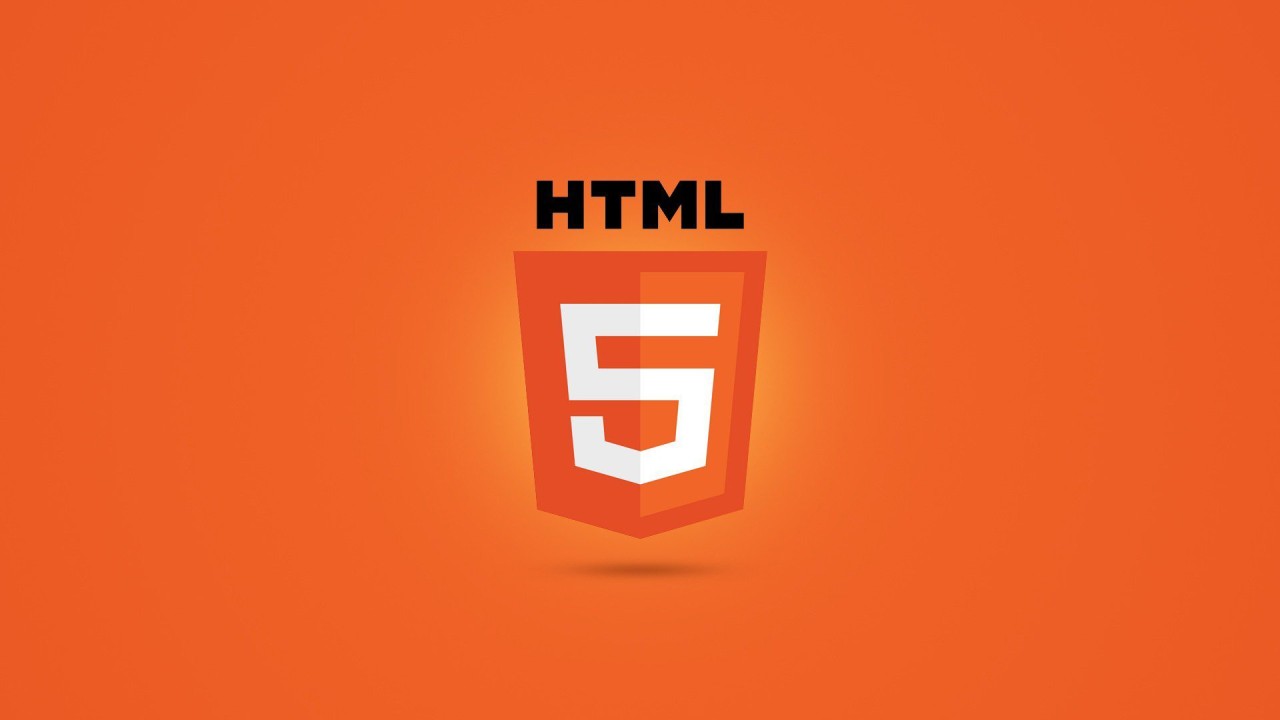
Introduction to <canvas>
The <canvas>
element in HTML5 is a powerful tool for rendering graphics, animations, and game elements directly in the browser. Unlike SVG, which is vector-based, Canvas provides a pixel-based rendering system, allowing for dynamic and interactive visuals.
Basic Syntax of <canvas>
To use the <canvas>
element, we define it in HTML and manipulate it using JavaScript:
<canvas id="myCanvas" width="500" height="400"></canvas> <script> let canvas = document.getElementById("myCanvas"); let ctx = canvas.getContext("2d"); </script>
Here, ctx
is the context object that provides methods for drawing on the canvas.
Drawing Shapes in Canvas
Canvas supports basic shapes such as rectangles, lines, circles, and custom paths.
Drawing Rectangles
ctx.fillStyle = "blue"; ctx.fillRect(50, 50, 150, 100); // (x, y, width, height)
This code draws a blue rectangle at (50,50) with a width of 150 and height of 100.
Drawing Lines
ctx.beginPath(); ctx.moveTo(20, 20); ctx.lineTo(180, 100); ctx.strokeStyle = "red"; ctx.lineWidth = 5; ctx.stroke();
This code creates a red diagonal line.
Drawing Circles
ctx.beginPath(); ctx.arc(200, 200, 50, 0, 2 * Math.PI); ctx.fillStyle = "green"; ctx.fill();
This code draws a filled green circle with a radius of 50 at (200, 200).
Custom Paths
ctx.beginPath(); ctx.moveTo(75, 50); ctx.lineTo(100, 75); ctx.lineTo(100, 25); ctx.closePath(); ctx.fillStyle = "purple"; ctx.fill();
This code creates a filled triangle.
Working with Colors & Gradients
Canvas allows filling shapes with colors, gradients, and patterns.
Solid Colors
ctx.fillStyle = "orange"; ctx.fillRect(10, 10, 100, 100);
Linear Gradient
let gradient = ctx.createLinearGradient(0, 0, 200, 0); gradient.addColorStop(0, "red"); gradient.addColorStop(1, "yellow"); ctx.fillStyle = gradient; ctx.fillRect(10, 10, 200, 100);
This creates a gradient from red to yellow.
Radial Gradient
let radGrad = ctx.createRadialGradient(100, 100, 10, 100, 100, 50); radGrad.addColorStop(0, "blue"); radGrad.addColorStop(1, "white"); ctx.fillStyle = radGrad; ctx.fillRect(50, 50, 150, 150);
This creates a circular gradient effect.
Animations in Canvas
Canvas animations involve continuously updating the canvas using the requestAnimationFrame()
method.
Basic Animation Example
let x = 0; function animate() { ctx.clearRect(0, 0, canvas.width, canvas.height); // Clear canvas ctx.fillStyle = "blue"; ctx.fillRect(x, 50, 50, 50); x += 2; if (x < canvas.width) { requestAnimationFrame(animate); } } animate();
This moves a blue square across the canvas.
Bouncing Ball Animation
let ball = { x: 50, y: 50, dx: 2, dy: 2, radius: 20 }; function drawBall() { ctx.beginPath(); ctx.arc(ball.x, ball.y, ball.radius, 0, Math.PI * 2); ctx.fillStyle = "red"; ctx.fill(); ctx.closePath(); } function updateBall() { ctx.clearRect(0, 0, canvas.width, canvas.height); drawBall(); ball.x += ball.dx; ball.y += ball.dy; if (ball.x + ball.radius > canvas.width || ball.x - ball.radius < 0) ball.dx *= -1; if (ball.y + ball.radius > canvas.height || ball.y - ball.radius < 0) ball.dy *= -1; requestAnimationFrame(updateBall); } updateBall();
This code creates a bouncing ball animation.
Conclusion
HTML5 Canvas provides a powerful way to create dynamic graphics, animations, and interactive content on the web. By mastering shapes, colors, gradients, and animations, you can develop engaging visuals for games, charts, and web applications. Keep experimenting to unlock its full potential!
Leave a Comment