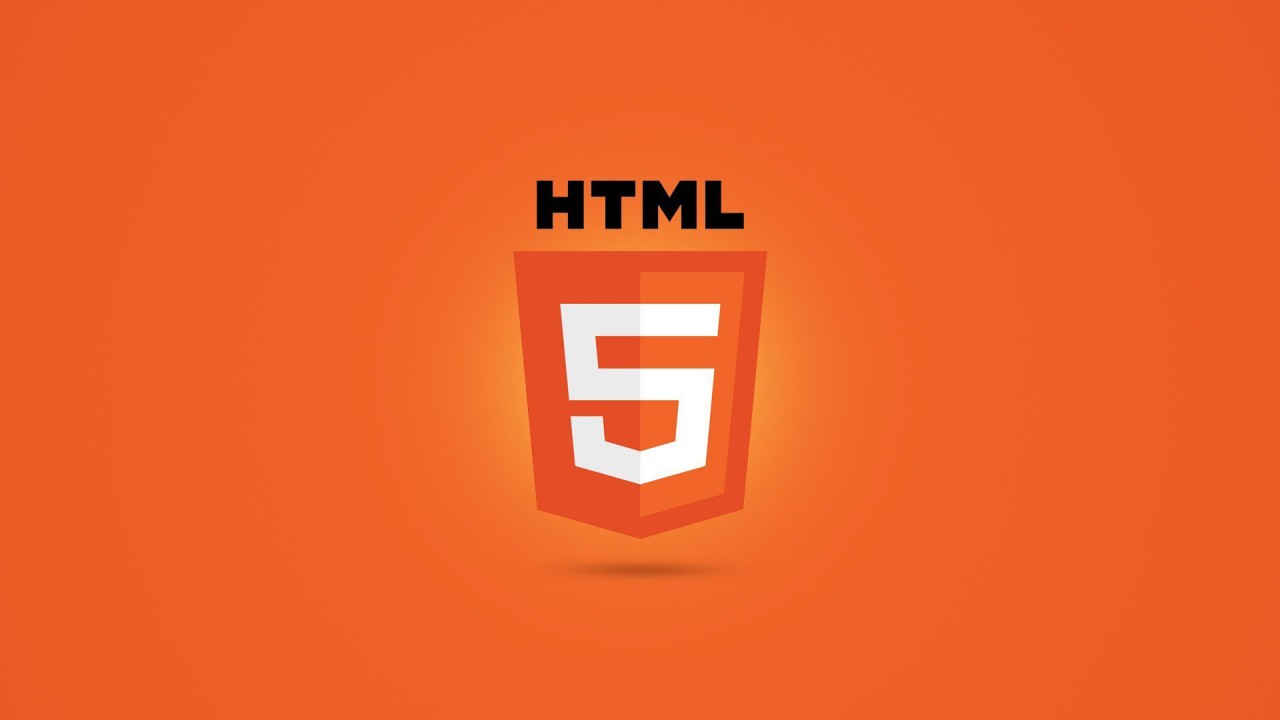
Forms are an essential part of web development, allowing users to input and submit data. In this article, we will explore advanced HTML form features, including form validation, autocomplete, datalist, and custom form controls.
1. Form Validation (Pattern Matching, Required Fields)
Form validation ensures that users enter data in the correct format before submission. HTML provides built-in validation attributes, reducing the need for JavaScript in many cases.
a) Required Fields
The required
attribute ensures that a field must be filled before submission.
<form> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <button type="submit">Submit</button> </form>
b) Pattern Matching
The pattern
attribute allows regex-based validation.
<form> <label for="phone">Phone Number (Format: 123-456-7890):</label> <input type="text" id="phone" name="phone" pattern="\d{3}-\d{3}-\d{4}" required> <button type="submit">Submit</button> </form>
If the format is incorrect, the browser will display an error message.
2. Autocomplete & Placeholder
The autocomplete
attribute enhances user experience by suggesting previously entered values, while placeholder
provides a hint inside input fields.
<form> <label for="email">Email:</label> <input type="email" id="email" name="email" autocomplete="on" placeholder="Enter your email"> <button type="submit">Submit</button> </form>
autocomplete="on"
enables auto-filling based on previous entries.placeholder="Enter your email"
displays text inside the input box.
3. Datalist (<datalist>
)
The <datalist>
element provides a dropdown of predefined options while allowing users to enter custom values.
<form> <label for="browser">Choose a browser:</label> <input list="browsers" id="browser" name="browser"> <datalist id="browsers"> <option value="Chrome"> <option value="Firefox"> <option value="Edge"> <option value="Safari"> <option value="Opera"> </datalist> <button type="submit">Submit</button> </form>
Users can either select from the options or type in their own value.
4. Custom Form Controls (Styling with CSS & JavaScript)
To enhance the look and functionality of forms, CSS and JavaScript can be used for custom styling and interactivity.
a) Styling Input Fields with CSS
input { width: 100%; padding: 10px; margin: 5px 0; border: 2px solid #007BFF; border-radius: 5px; } input:focus { border-color: #0056b3; outline: none; }
b) Custom File Input with JavaScript
<form> <label for="file">Upload File:</label> <input type="file" id="file" hidden> <button type="button" onclick="document.getElementById('file').click();">Choose File</button> <span id="file-name">No file chosen</span> </form> <script> document.getElementById('file').addEventListener('change', function() { document.getElementById('file-name').textContent = this.files[0].name; }); </script>
This script allows users to click a button instead of the default file input and displays the chosen file name.
Conclusion
HTML provides powerful built-in form features like validation, autocomplete, and datalist to enhance usability. With CSS and JavaScript, forms can be further customized for a better user experience. Mastering these techniques will help you create intuitive and user-friendly forms for your web applications.
Want to take your form skills further? Try integrating JavaScript form validation and custom UI components using frameworks like Bootstrap or Tailwind CSS!
Leave a Comment