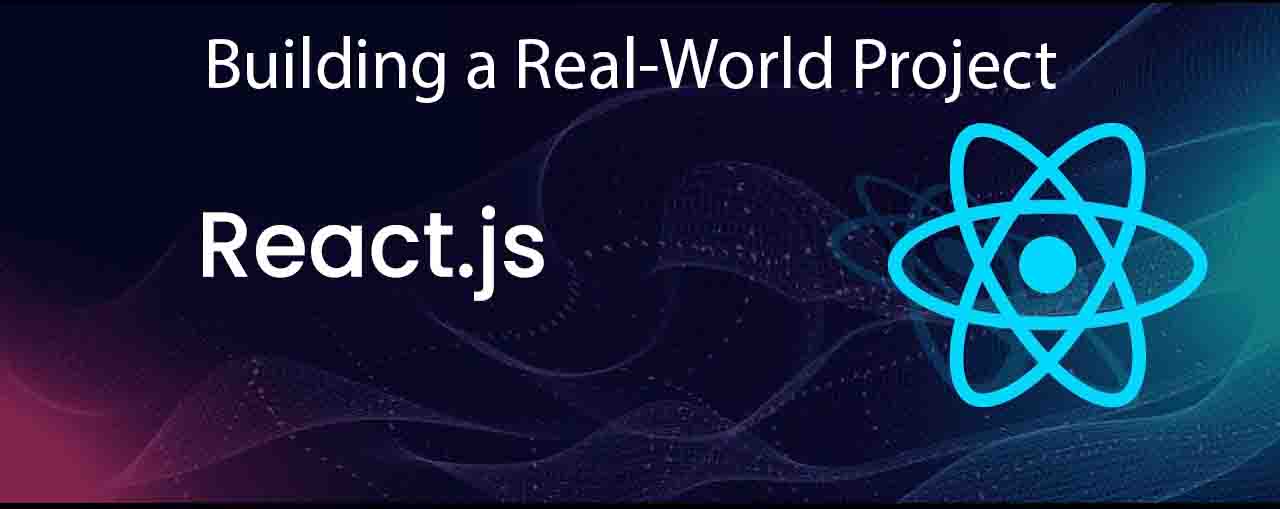
Building a Real-World Project with React
Creating a real-world application with React requires careful planning, systematic implementation, and an understanding of deployment processes. This article will guide you through the following steps:
- Designing the application architecture.
- Implementing key features step by step.
- Deploying the application.
Designing the Application Architecture
Before writing any code, it is crucial to design an architecture that defines the structure and flow of your application. A well-designed architecture ensures scalability, maintainability, and clarity.
Steps for Designing Application Architecture
- Understand the Requirements:
- Identify the primary features of the application.
- Define user roles and their interactions with the application.
- Choose the Tech Stack:
- Frontend: React (with libraries like Redux, React Router, or Tailwind CSS).
- Backend: Node.js, Express, or any other preferred backend.
- Database: MongoDB, PostgreSQL, or Firebase for real-time apps.
- Break Down Features into Components: Divide the UI into reusable React components based on functionality. For example:
- Header
- Footer
- Sidebar
- Feature-specific components (e.g., LoginForm, ProductList, CartItem).
- Define State Management Strategy: Decide how data will flow through the application. Options include:
- React Context API for simple applications.
- Redux Toolkit for complex state management.
- Plan API Integration: Design RESTful or GraphQL APIs for backend communication. Ensure endpoints are documented for easier integration.
- Set Up Routing: Use React Router to define navigation paths and dynamic routes.
Example Architecture Diagram:
App ├ Header ├ Sidebar ├ MainContent ├ Home ├ ProductList ├ ProductDetails ├ Footer
Implementing Key Features Step by Step
Implementation involves coding the planned features and components while ensuring code reusability and best practices.
Step 1: Set Up the React Project
- Initialize the Project:
npx create-react-app my-app cd my-app
- Install Dependencies:
npm install react-router-dom redux @reduxjs/toolkit axios tailwindcss
- Set Up Folder Structure:
src ├ components ├ pages ├ redux ├ utils
Step 2: Implement Core Features
Feature 1: User Authentication
- Create a Login Form:
const LoginForm = () => { const handleLogin = (e) => { e.preventDefault(); // Add login logic here }; return ( <form onSubmit={handleLogin}> <input type="text" placeholder="Username" required /> <input type="password" placeholder="Password" required /> <button type="submit">Login</button> </form> ); }; export default LoginForm;
- Handle Authentication State: Use Context API or Redux to manage the user's login state.
Feature 2: Product List and Details
- Fetch Data from an API:
import { useEffect, useState } from 'react'; import axios from 'axios'; const ProductList = () => { const [products, setProducts] = useState([]); useEffect(() => { axios.get('/api/products').then((response) => setProducts(response.data)); }, []); return ( <ul> {products.map((product) => ( <li key={product.id}>{product.name}</li> ))} </ul> ); }; export default ProductList;
- Display Product Details: Use React Router for dynamic routes:
import { useParams } from 'react-router-dom'; const ProductDetails = () => { const { id } = useParams(); const [product, setProduct] = useState(null); useEffect(() => { // Fetch product details by ID }, [id]); return product ? <div>{product.name}</div> : <p>Loading...</p>; }; export default ProductDetails;
Feature 3: Shopping Cart
- Create a Cart Component: Manage cart state using Redux.
import { useSelector, useDispatch } from 'react-redux'; const Cart = () => { const cart = useSelector((state) => state.cart.items); const dispatch = useDispatch(); const removeFromCart = (id) => { dispatch({ type: 'cart/removeItem', payload: id }); }; return ( <ul> {cart.map((item) => ( <li key={item.id}> {item.name} <button onClick={() => removeFromCart(item.id)}>Remove</button> </li> ))} </ul> ); }; export default Cart;
Deploying the Application
Deployment is the final step to make your application accessible to users.
Step 1: Build the Application
Generate an optimised production build:
npm run build
Step 2: Choose a Hosting Platform
Popular options include:
- Netlify: Ideal for static React apps.
- Vercel: Perfect for modern frameworks.
- AWS Amplify: Provides scalability and flexibility.
- Firebase Hosting: Offers real-time database integration.
Step 3: Deploy the Build
For example, using Netlify:
- Drag and drop the
build
folder into the Netlify dashboard. - Configure build settings if using CI/CD pipelines.
For example, using Vercel:
- Install the Vercel CLI:
npm install -g vercel
- Deploy the project:
vercel
Step 4: Monitor and Maintain
Use tools like Google Analytics or Sentry to monitor app performance and errors post-deployment.
Conclusion
Building a real-world React application involves thoughtful architecture design, structured implementation, and seamless deployment. By following the steps outlined in this article, you can create a scalable and maintainable React application, ready to serve users in the real world.
Leave a Comment