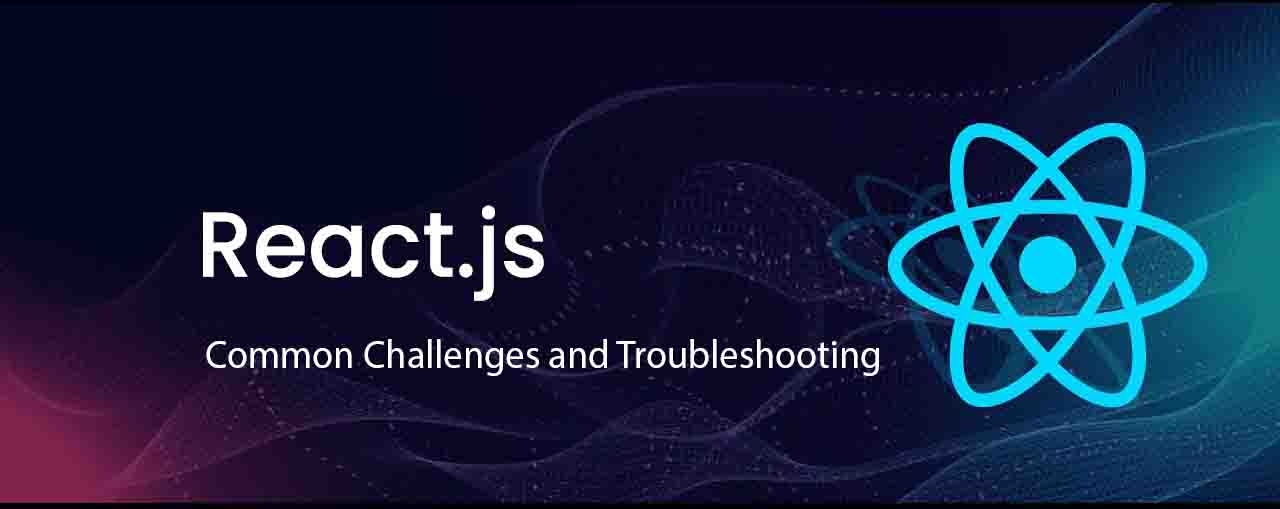
Common Challenges and Troubleshooting in React
Developing applications in React can come with its own set of challenges. From debugging issues to handling common errors, understanding the pitfalls and best practices can save time and frustration. This article addresses:
- Debugging techniques.
- Solving common React errors.
- Tips for avoiding pitfalls.
Debugging Techniques
Debugging is an essential part of development. Using the right tools and methods can streamline the process and help identify issues efficiently.
1. Using Browser Developer Tools
Modern browsers provide excellent developer tools for inspecting and debugging React applications:
- Inspect Element: Right-click on any element in your application to inspect its structure and styles.
- Console Tab: Check for JavaScript errors and warnings.
- Network Tab: Monitor API requests, responses, and loading times.
2. React Developer Tools Extension
Install the React Developer Tools extension for Chrome or Firefox to:
- Inspect the component tree and its state/props.
- View the re-rendering behaviour of components.
- Debug issues related to props drilling and context.
3. Debugging with console.log
Strategic placement of console.log
statements can help trace issues. Use it sparingly to log:
- Function arguments.
- Component states and props.
- API response data.
4. Breakpoints in Code Editors
Modern IDEs like Visual Studio Code allow you to:
- Set breakpoints in your code to pause execution.
- Inspect variables and stack traces.
- Step through the code line by line.
5. Error Boundaries
Use React’s Error Boundaries to catch JavaScript errors in the component tree.
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } componentDidCatch(error, info) { console.error("Error caught in ErrorBoundary:", error, info); } render() { if (this.state.hasError) { return <h1>Something went wrong.</h1>; } return this.props.children; } } export default ErrorBoundary;
Wrap components with ErrorBoundary
to handle uncaught errors.
Solving Common React Errors
Here are some frequently encountered errors in React and how to resolve them:
1. Error: "Cannot read property 'X' of undefined"
Cause:
Attempting to access a property of an undefined variable.
Solution:
- Check if the variable is initialized before accessing its properties.
- Use optional chaining (
?.
) to avoid runtime errors:
console.log(data?.property);
2. Error: "Invalid Hook Call"
Cause:
- Using React hooks incorrectly (e.g., outside a functional component or custom hook).
- Multiple versions of React in the
node_modules
folder.
Solution:
- Ensure hooks are used only in functional components or custom hooks.
- Check for duplicate React versions:
npm ls react
- Reinstall dependencies if necessary.
3. Error: "Each child in a list should have a unique 'key' prop"
Cause:
React requires unique key
props for list items to optimise rendering.
Solution:
Add a unique key
to each child:
<ul> {items.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul>
4. Error: "Maximum update depth exceeded"
Cause:
Infinite re-renders caused by improper state updates or side effects.
Solution:
- Avoid updating state directly within
useEffect
without conditions:
useEffect(() => { setCount(count + 1); // Avoid this pattern }, [count]);
- Add appropriate dependencies to the dependency array.
Tips for Avoiding Pitfalls
1. Optimise State Management
- Minimise state in components. Only manage what is necessary.
- Use libraries like Redux Toolkit or Zustand for global state management in large applications.
2. Avoid Over-Rendering
- Use
React.memo
to prevent unnecessary re-renders of child components. - Optimise functional components with
useCallback
anduseMemo
.
3. Clean Up Side Effects
Always clean up effects to avoid memory leaks:
useEffect(() => { const timer = setInterval(() => { console.log("Running"); }, 1000); return () => clearInterval(timer); // Cleanup }, []);
4. Follow Coding Standards
- Name files and components consistently (e.g., PascalCase for components).
- Use TypeScript to catch errors early.
5. Test Components Thoroughly
- Write unit tests with Jest and React Testing Library.
- Test edge cases to avoid runtime issues.
Conclusion
Debugging and troubleshooting React applications can be straightforward if you follow systematic approaches. By mastering debugging tools, understanding common errors, and adopting best practices, you can build robust and error-free React applications.
Leave a Comment