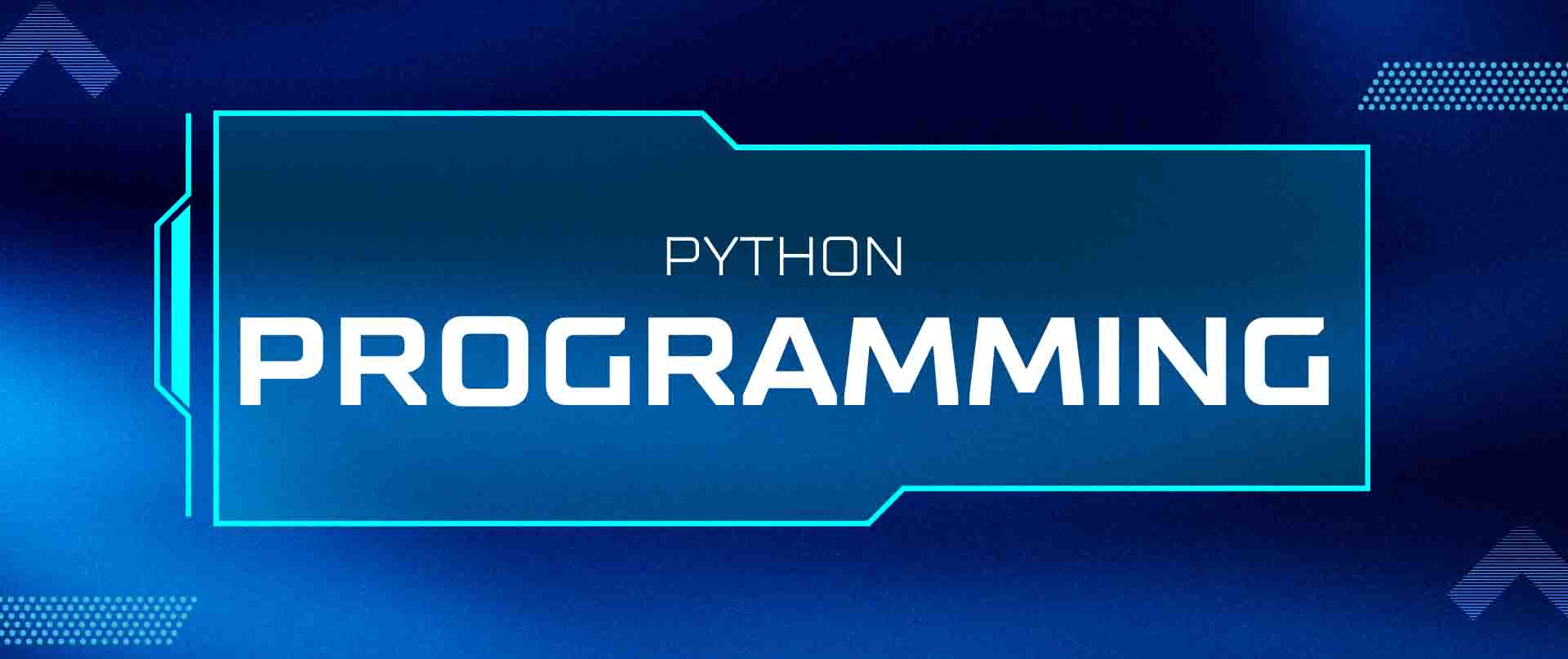
Python is one of the most popular programming languages today, known for its readability, simplicity, and versatility. Whether you're a beginner or a seasoned developer, following best practices in Python development ensures that your code remains clean, efficient, and maintainable. In this blog, we’ll explore Python best practices, resources for further learning, and ways to build real-world projects.
Best Practices in Python Development
Adhering to best practices is essential for writing high-quality Python code. Here are some of the key principles that can guide your Python development process:
1. Follow PEP 8 (Python Enhancement Proposals)
PEP 8 is the official style guide for Python code. It outlines conventions for writing clean, readable, and consistent Python code. Some important aspects include:
- Indentation: Use 4 spaces per indentation level.
- Line Length: Limit all lines to a maximum of 79 characters.
- Naming Conventions: Use lowercase words separated by underscores for function and variable names (
snake_case
), and capitalize class names (CamelCase
). - Whitespace: Avoid extra spaces at the beginning or end of lines and ensure spaces around operators and after commas.
2. Write Readable and Self-Documenting Code
Python is designed to be readable, so make your code as clear as possible. Use descriptive variable and function names that convey the purpose of the code. Write docstrings for functions, classes, and modules to explain their purpose and usage.
python CopyEdit def calculate_area(radius: float) -> float: """ Calculate the area of a circle. Parameters: radius (float): The radius of the circle. Returns: float: The area of the circle. """ return 3.14 * radius ** 2
3. Use List Comprehensions and Generator Expressions
List comprehensions and generator expressions are more Pythonic and concise ways to create lists or other iterables. They not only make your code more readable but also improve performance.
List Comprehension:
python CopyEdit squares = [x**2 for x in range(10)]
Generator Expression:
python CopyEdit squares_gen = (x**2 for x in range(10))
Generator expressions are more memory efficient as they yield values one at a time instead of generating a whole list.
4. Leverage Python’s Standard Library
Python’s standard library is vast and includes modules for regular expressions, file handling, data serialization, networking, and more. Before reinventing the wheel, check the library to see if there’s an existing solution. This can save time and effort.
5. Handle Exceptions Properly
Avoid catching general exceptions unless necessary. Handle specific exceptions to provide better error messages and avoid masking issues.
python CopyEdit try: num = int(input("Enter a number: ")) except ValueError: print("That's not a valid number.")
6. Use Virtual Environments
Virtual environments allow you to manage dependencies for each project separately. This is particularly important for avoiding conflicts between different project dependencies.
To create and activate a virtual environment:
bash CopyEdit python -m venv myenv source myenv/bin/activate # On Windows use myenv\Scripts\activate
7. Optimize Code for Performance
While Python is generally slower than languages like C or Java, you can still make your code more efficient:
- Avoid using excessive global variables.
- Minimize unnecessary loops and function calls.
- Use built-in data structures (like sets, dictionaries, and tuples) whenever possible.
- Profile your code using tools like
cProfile
and optimize performance bottlenecks.
8. Testing and Debugging
Test your code to catch errors early. Python has several testing libraries, such as unittest
, pytest
, and nose
. Write unit tests for your functions to verify that they work as expected. Additionally, use the built-in pdb
debugger for diagnosing issues.
Example of writing a simple unit test with unittest
:
python CopyEdit import unittest class TestMathOperations(unittest.TestCase): def test_add(self): self.assertEqual(add(1, 2), 3) if __name__ == '__main__': unittest.main()
Resources for Further Learning
As Python is a vast language, continuous learning is essential to stay up-to-date with its evolving features and libraries. Here are some excellent resources to further enhance your Python skills:
1. Official Python Documentation
The official Python documentation is the best place to start for any Python-related query. It provides comprehensive guides, references, and tutorials for all aspects of Python programming.
Leave a Comment