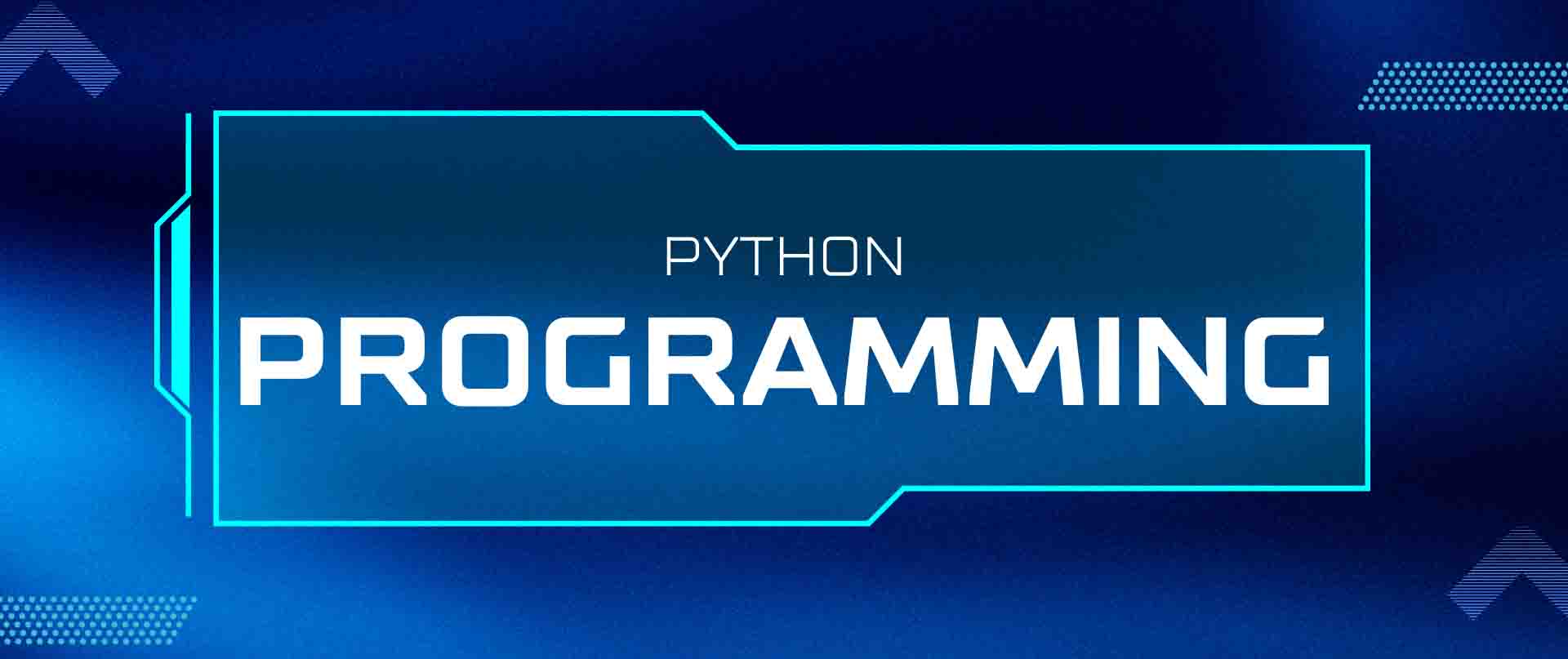
Python is a powerful and versatile programming language that offers several advanced features to enhance performance, maintainability, and efficiency. In this article, we will explore three critical advanced Python topics:
- Multithreading and Concurrency
- Metaprogramming and Decorators
- Using Asyncio for Asynchronous Programming
1. Multithreading and Concurrency
Understanding Concurrency
Concurrency allows multiple tasks to be executed seemingly at the same time. In Python, concurrency can be achieved using multithreading and multiprocessing. However, Python’s Global Interpreter Lock (GIL) limits true parallel execution in multithreading, making it more suitable for I/O-bound tasks rather than CPU-bound tasks.
Multithreading in Python
Multithreading involves using the threading
module to run multiple threads concurrently. Each thread runs independently and can perform I/O operations without blocking the entire program.
import threading import time def print_numbers(): for i in range(5): time.sleep(1) print(f"Number: {i}") thread1 = threading.Thread(target=print_numbers) thread1.start() print("Thread started") thread1.join() print("Thread finished")
When to Use Multithreading
- Performing I/O operations such as reading/writing files, network requests, and database operations.
- When tasks are independent and can be executed concurrently.
Multiprocessing for True Parallelism
For CPU-bound tasks, the multiprocessing
module is a better alternative as it creates separate processes, bypassing the GIL.
import multiprocessing def compute(): print("Processing...") process = multiprocessing.Process(target=compute) process.start() process.join()
2. Metaprogramming and Decorators
What is Metaprogramming?
Metaprogramming is the ability of a program to modify or generate other parts of the program at runtime. In Python, metaprogramming is often done using decorators, metaclasses, and reflection.
Understanding Decorators
Decorators are functions that modify the behavior of other functions without changing their structure. They are widely used in frameworks and libraries.
Example of a Simple Decorator:
def my_decorator(func): def wrapper(): print("Before function execution") func() print("After function execution") return wrapper @my_decorator def say_hello(): print("Hello, World!") say_hello()
Built-in Python Decorators
@staticmethod
– Defines a static method within a class.@classmethod
– Defines a class method that operates on the class rather than instances.@property
– Converts a method into a read-only property.
Example:
class Example: def __init__(self, value): self._value = value @property def value(self): return self._value obj = Example(10) print(obj.value) # 10
3. Using Asyncio for Asynchronous Programming
What is Asyncio?
asyncio
is a Python library used for writing concurrent code using the async/await syntax. It is particularly useful for I/O-bound operations such as networking and database interactions.
Writing Asynchronous Code with Async/Await
import asyncio async def say_hello(): await asyncio.sleep(2) print("Hello, Asyncio!") async def main(): await say_hello() asyncio.run(main())
Running Multiple Async Tasks Concurrently
Using asyncio.gather()
, multiple async tasks can be executed concurrently.
async def task1(): await asyncio.sleep(1) print("Task 1 completed") async def task2(): await asyncio.sleep(2) print("Task 2 completed") async def main(): await asyncio.gather(task1(), task2()) asyncio.run(main())
When to Use Asyncio
- Networking applications (e.g., web scraping, API calls).
- Handling multiple I/O-bound operations efficiently.
- Running multiple tasks that require non-blocking execution.
Conclusion
These advanced Python concepts—multithreading and concurrency, metaprogramming with decorators, and asyncio for asynchronous programming—can significantly enhance your Python applications. By mastering these techniques, you can build more efficient, scalable, and maintainable software solutions.
Leave a Comment