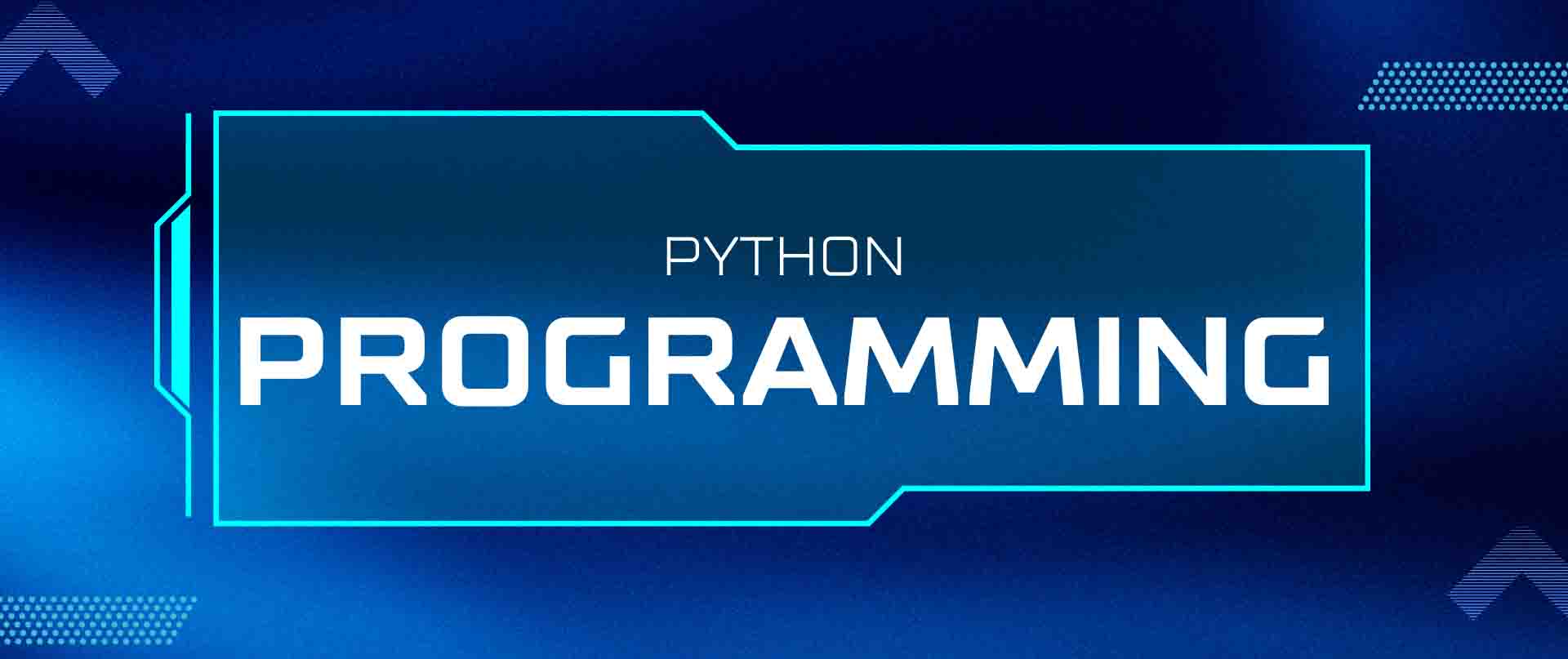
APIs (Application Programming Interfaces) are essential for enabling communication between different software applications. In this guide, we will explore RESTful API concepts, how to create APIs using Flask-RESTful, and how to consume APIs using the Requests library in Python.
Understanding RESTful API Concepts
A RESTful API (Representational State Transfer) is an architectural style that allows clients to interact with a server using HTTP methods. The key principles of RESTful APIs include:
- Statelessness: Each request from the client contains all the information the server needs to fulfill it.
- Client-Server Architecture: The client and server operate independently.
- Uniform Interface: Standardized API endpoints and methods (GET, POST, PUT, DELETE).
- Resource-Based Design: API endpoints represent resources (e.g.,
/users
,/products
). - Use of HTTP Methods:
GET
: Retrieve data.POST
: Create new data.PUT/PATCH
: Update existing data.DELETE
: Remove data.
Creating APIs Using Flask-RESTful
Installing Flask-RESTful
First, install the required libraries:
pip install flask flask-restful
Setting Up a Simple API
Create a new Python file, app.py
, and add the following:
from flask import Flask, request from flask_restful import Resource, Api app = Flask(__name__) api = Api(app) # In-memory data storage (for demonstration) users = {} # User Resource class User(Resource): def get(self, user_id): if user_id in users: return users[user_id], 200 return {"message": "User not found"}, 404 def post(self, user_id): users[user_id] = request.json return users[user_id], 201 def put(self, user_id): if user_id in users: users[user_id].update(request.json) return users[user_id], 200 return {"message": "User not found"}, 404 def delete(self, user_id): if user_id in users: del users[user_id] return {"message": "User deleted"}, 200 return {"message": "User not found"}, 404 # Add Resource to API api.add_resource(User, "/user/<string:user_id>") if __name__ == "__main__": app.run(debug=True)
Running the API
Run the script:
python app.py
Your API will be available at http://127.0.0.1:5000
.
Consuming APIs Using Requests
The requests
library makes it easy to interact with APIs. Install it using:
pip install requests
Making API Calls
Create a client.py
file and add:
import requests BASE_URL = "http://127.0.0.1:5000/user/" # Create a user response = requests.post(BASE_URL + "1", json={"name": "John Doe", "age": 30}) print(response.json()) # Retrieve user data response = requests.get(BASE_URL + "1") print(response.json()) # Update user data response = requests.put(BASE_URL + "1", json={"age": 31}) print(response.json()) # Delete user response = requests.delete(BASE_URL + "1") print(response.json())
Expected Output
{'name': 'John Doe', 'age': 30} {'name': 'John Doe', 'age': 30} {'name': 'John Doe', 'age': 31} {'message': 'User deleted'}
Conclusion
In this guide, we covered:
- The fundamentals of RESTful API design.
- How to build a RESTful API using Flask-RESTful.
- How to consume APIs using the Requests library.
This knowledge allows you to develop and integrate APIs efficiently in Python applications. Happy coding!
Leave a Comment