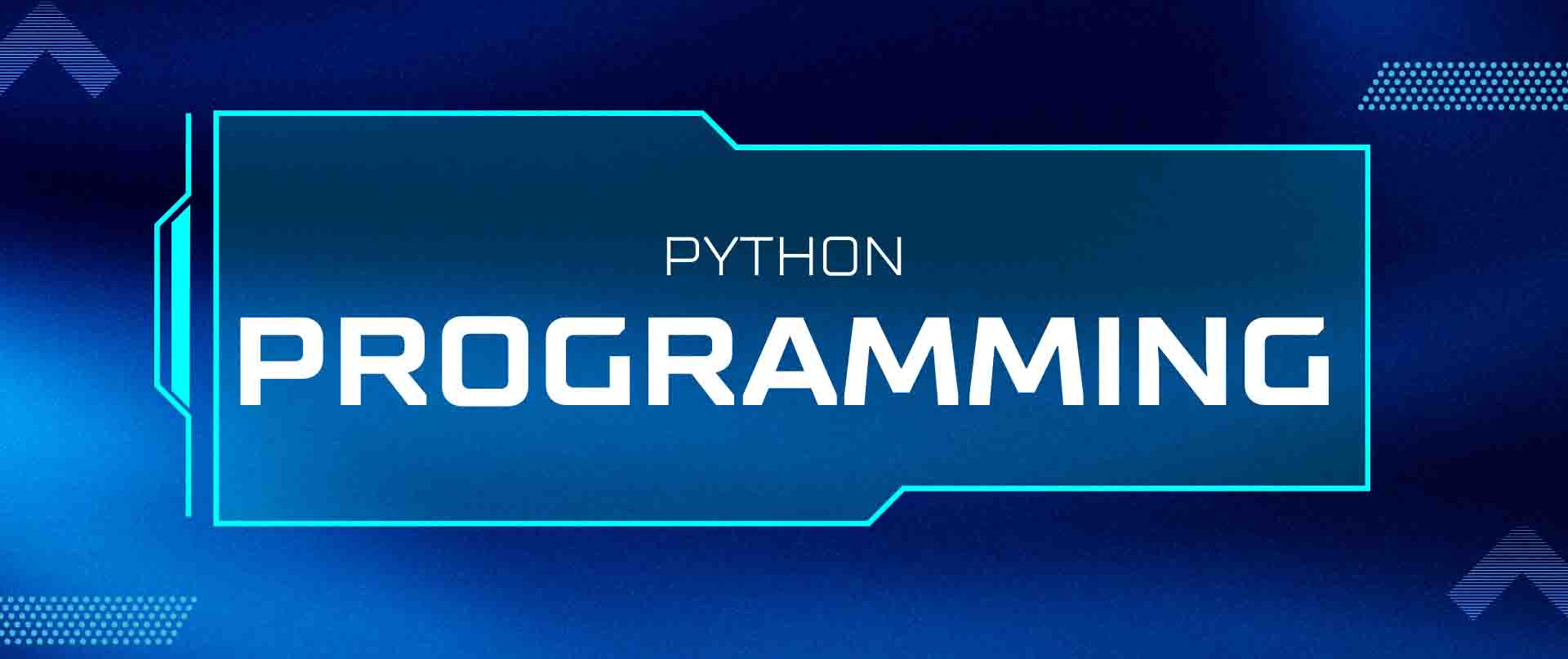
Software testing is a crucial part of the development lifecycle, ensuring that applications function correctly and meet requirements. Python provides robust testing frameworks like unittest
and pytest
, alongside effective debugging and logging tools. This guide explores how to write unit tests, automate testing with pytest
, and utilize debugging and logging techniques.
1. Writing Unit Tests with unittest
Introduction to unittest
Python's built-in unittest
module provides a framework for writing and running tests. It follows the xUnit style, similar to JUnit in Java.
Setting Up a Unit Test
A unit test isolates and verifies the behavior of a specific function or method. Here’s a simple example:
import unittest # Function to be tested def add(a, b): return a + b class TestMathOperations(unittest.TestCase): def test_add(self): self.assertEqual(add(2, 3), 5) self.assertEqual(add(-1, 1), 0) self.assertEqual(add(0, 0), 0) if __name__ == '__main__': unittest.main()
Key Features of unittest
assertEqual(a, b)
: Checks ifa
is equal tob
assertTrue(x)
: Checks ifx
isTrue
assertFalse(x)
: Checks ifx
isFalse
setUp()
andtearDown()
: Used to prepare and clean up test environments
2. Test Automation with pytest
Why Use pytest
?
pytest
is a powerful testing framework that simplifies writing and running tests. It offers:
- Less boilerplate compared to
unittest
- Enhanced test discovery
- Fixtures for setup and teardown
Installing pytest
pip install pytest
Writing a Test with pytest
# Function to be tested def multiply(a, b): return a * b # Test case def test_multiply(): assert multiply(2, 3) == 6 assert multiply(-1, 1) == -1 assert multiply(0, 10) == 0
Running Tests
Save the test file (e.g., test_math.py
) and execute:
pytest test_math.py
Using Fixtures in pytest
Fixtures help manage test setup and teardown.
import pytest @pytest.fixture def sample_data(): return {'a': 5, 'b': 10} def test_addition(sample_data): assert sample_data['a'] + sample_data['b'] == 15
3. Debugging and Logging in Python
Debugging with pdb
The pdb
module is Python's built-in debugger. You can set breakpoints using:
import pdb def divide(a, b): pdb.set_trace() return a / b divide(10, 2)
Commands within pdb
:
n
(next): Execute the next lines
(step): Step into a functionc
(continue): Resume executionq
(quit): Exit debugger
Logging in Python
Logging is essential for tracking application behavior. Use Python’s logging
module for structured logs.
Basic Logging Setup
import logging logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s') logging.info("Application started") logging.warning("Low disk space") logging.error("File not found")
Logging to a File
logging.basicConfig(filename='app.log', level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s') logging.debug("Debugging message")
Conclusion
Software testing in Python is streamlined using unittest
and pytest
, while debugging and logging enhance maintainability. By integrating these techniques, developers can ensure robust and error-free applications.
Leave a Comment