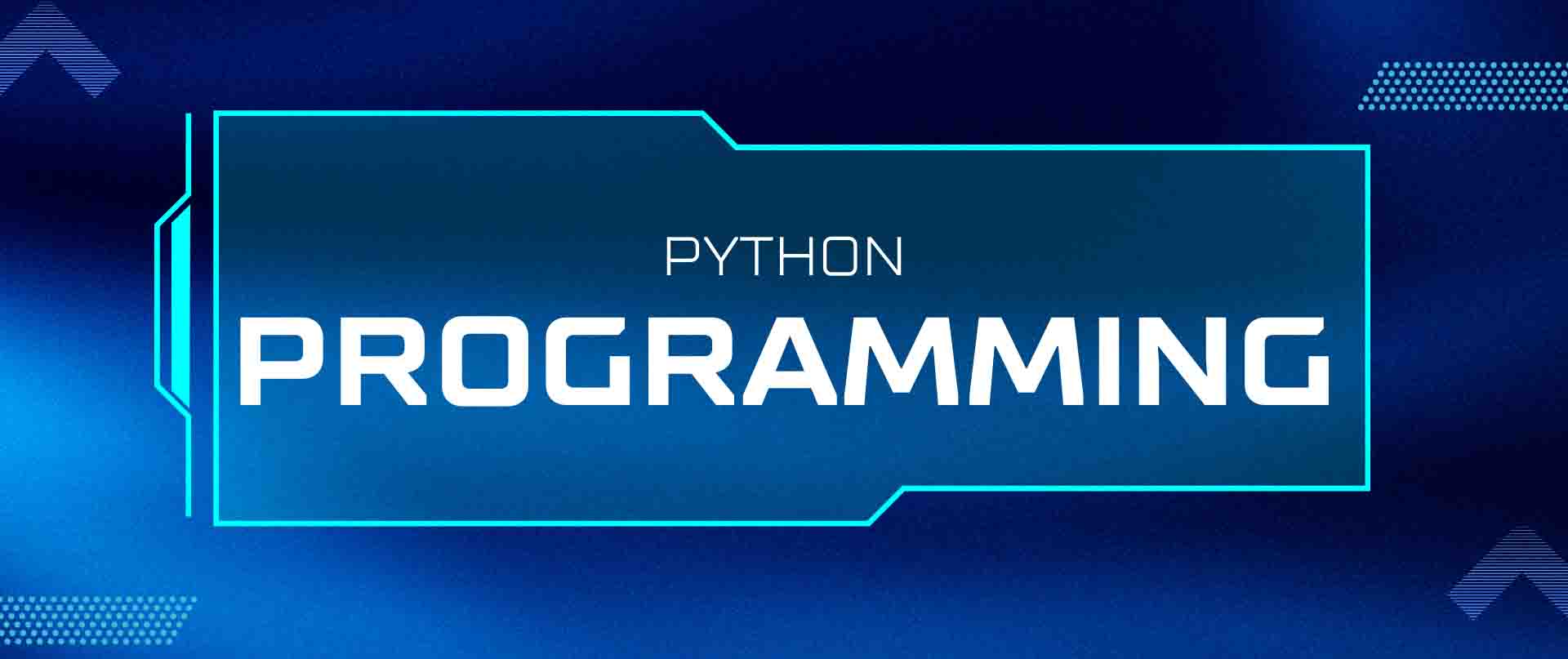
Introduction
Python has become the leading language for data science and machine learning due to its rich ecosystem of libraries. This blog provides a comprehensive guide to using NumPy, Pandas, Matplotlib, Seaborn, and Scikit-Learn to manipulate data, visualize trends, and build machine learning models.
1. Introduction to NumPy & Pandas
NumPy: The Foundation of Scientific Computing
NumPy (Numerical Python) is a fundamental library for numerical operations in Python. It provides support for multi-dimensional arrays, mathematical functions, and efficient computation.
Key Features of NumPy:
- N-dimensional array object (ndarray)
- Fast mathematical operations
- Broadcasting support
- Linear algebra and random number generation
Example: Creating a NumPy Array
import numpy as np # Creating an array a = np.array([1, 2, 3, 4, 5]) print(a)
Pandas: Data Manipulation Made Easy
Pandas is a powerful library for data analysis and manipulation. It introduces DataFrames, which allow for efficient handling of structured data.
Key Features of Pandas:
- DataFrame and Series objects
- Handling missing data
- Data filtering, grouping, and merging
- Importing and exporting data (CSV, Excel, SQL, JSON)
Example: Creating a Pandas DataFrame
import pandas as pd # Creating a DataFrame data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) print(df)
2. Data Visualization with Matplotlib & Seaborn
Data visualization is crucial for understanding patterns and trends in data.
Matplotlib: The Standard Visualization Library
Matplotlib allows users to create line charts, bar plots, histograms, scatter plots, and more.
Example: Creating a Simple Line Plot
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [10, 20, 25, 30, 40] plt.plot(x, y, marker='o', linestyle='-', color='b') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Simple Line Plot') plt.show()
Seaborn: Statistical Data Visualization
Seaborn is built on top of Matplotlib and provides an aesthetically pleasing and high-level interface for drawing attractive statistical graphs.
Example: Creating a Seaborn Histogram
import seaborn as sns import numpy as np # Generating random data data = np.random.randn(1000) # Creating the histogram sns.histplot(data, kde=True, bins=30, color='blue') plt.show()
3. Introduction to Machine Learning with Scikit-Learn
Scikit-Learn is the most widely used library for machine learning in Python. It provides tools for data preprocessing, model training, and evaluation.
Key Features of Scikit-Learn:
- Supervised learning (Regression, Classification)
- Unsupervised learning (Clustering, Dimensionality Reduction)
- Model evaluation and selection
- Feature extraction and engineering
Example: Training a Simple Linear Regression Model
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error import numpy as np # Generating synthetic data X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1) y = np.array([10, 20, 30, 40, 50]) # Splitting the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Training the model model = LinearRegression() model.fit(X_train, y_train) # Making predictions y_pred = model.predict(X_test) # Evaluating the model mse = mean_squared_error(y_test, y_pred) print(f'Mean Squared Error: {mse}')
Conclusion
Python’s data science ecosystem is rich and powerful. NumPy and Pandas help manipulate and analyze data, Matplotlib and Seaborn enhance visualization, and Scikit-Learn provides the tools needed to develop machine learning models. By mastering these libraries, you can unlock the full potential of data science and machine learning.
Are you ready to take the next step in your data science journey? Start experimenting with real-world datasets and enhance your skills!
Leave a Comment