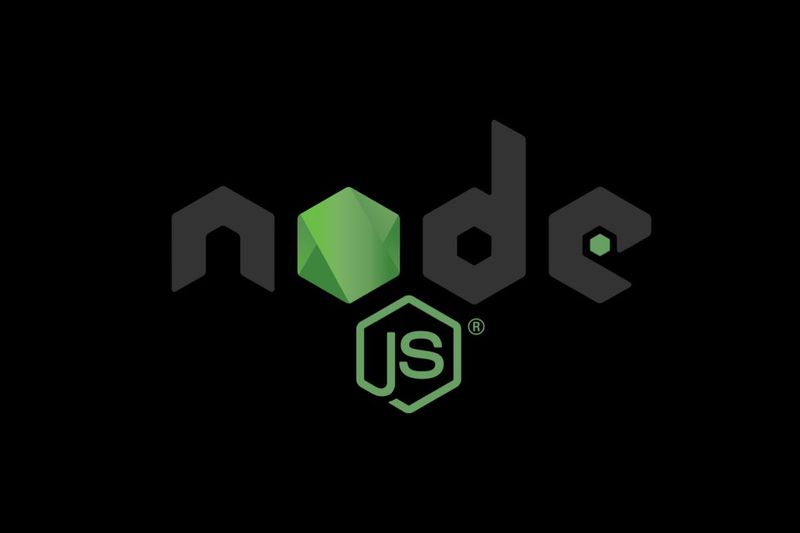
Working with Databases in Node.js: A Comprehensive Guide
Introduction to Databases in Node.js
Databases are a crucial component of modern web applications, enabling the storage, retrieval, and management of data efficiently. In the Node.js ecosystem, developers have a variety of database choices, but two of the most popular are MongoDB (a NoSQL database) and PostgreSQL (a relational SQL database). To interact with these databases, we commonly use Mongoose (for MongoDB) and Sequelize (for PostgreSQL).
This guide will cover:
• Connecting Node.js to MongoDB using Mongoose
• Performing CRUD operations with MongoDB
• Connecting to PostgreSQL using Sequelize
• Performing CRUD operations with PostgreSQL
Let's get started!
________________________________________
Connecting to MongoDB with Mongoose
What is MongoDB?
MongoDB is a NoSQL database that stores data in JSON-like documents. It is highly scalable and flexible, making it a great choice for modern applications.
What is Mongoose?
Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It provides schema validation and easy-to-use methods for working with MongoDB.
Installing MongoDB and Mongoose
Before we begin, make sure you have MongoDB installed on your machine or use a cloud service like MongoDB Atlas. Then, install Mongoose in your Node.js project:
npm install mongoose
Connecting to MongoDB
Create a db.js file and set up the connection:
const mongoose = require('mongoose');
const connectDB = async () => {
try {
await mongoose.connect('mongodb://localhost:27017/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
console.log('MongoDB Connected Successfully');
} catch (error) {
console.error('Error connecting to MongoDB', error);
process.exit(1);
}
};
module.exports = connectDB;
Then, call this function in your index.js file:
const express = require('express');
const connectDB = require('./db');
const app = express();
connectDB();
app.listen(3000, () => console.log('Server running on port 3000'));
________________________________________
CRUD Operations with MongoDB
Let's define a Mongoose model for a User collection:
const mongoose = require('mongoose');
const UserSchema = new mongoose.Schema({
name: String,
email: String,
age: Number,
});
const User = mongoose.model('User', UserSchema);
module.exports = User;
Creating a New User
const User = require('./models/User');
const createUser = async () => {
const newUser = new User({ name: 'John Doe', email: 'john@example.com', age: 25 });
await newUser.save();
console.log('User created:', newUser);
};
createUser();
Reading Users
const getUsers = async () => {
const users = await User.find();
console.log('Users:', users);
};
getUsers();
Updating a User
const updateUser = async (id) => {
await User.findByIdAndUpdate(id, { age: 30 });
console.log('User updated');
};
Deleting a User
const deleteUser = async (id) => {
await User.findByIdAndDelete(id);
console.log('User deleted');
};
________________________________________
Using PostgreSQL with Sequelize
What is PostgreSQL?
PostgreSQL is a powerful, open-source relational database system that supports SQL queries and complex transactions.
What is Sequelize?
Sequelize is a promise-based ORM for Node.js that makes it easier to work with SQL databases like PostgreSQL.
Installing PostgreSQL and Sequelize
Ensure you have PostgreSQL installed and then install Sequelize and the PostgreSQL driver:
npm install sequelize pg pg-hstore
Connecting to PostgreSQL
Create a db.js file:
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('mydatabase', 'username', 'password', {
host: 'localhost',
dialect: 'postgres',
});
const connectDB = async () => {
try {
await sequelize.authenticate();
console.log('PostgreSQL Connected Successfully');
} catch (error) {
console.error('Error connecting to PostgreSQL', error);
}
};
module.exports = { sequelize, connectDB };
Then, call this function in index.js:
const express = require('express');
const { connectDB } = require('./db');
const app = express();
connectDB();
app.listen(3000, () => console.log('Server running on port 3000'));
________________________________________
CRUD Operations with PostgreSQL
Define a User model:
const { Sequelize, DataTypes } = require('sequelize');
const { sequelize } = require('./db');
const User = sequelize.define('User', {
name: { type: DataTypes.STRING, allowNull: false },
email: { type: DataTypes.STRING, unique: true, allowNull: false },
age: { type: DataTypes.INTEGER },
});
sequelize.sync();
module.exports = User;
Creating a User
const createUser = async () => {
const newUser = await User.create({ name: 'Jane Doe', email: 'jane@example.com', age: 28 });
console.log('User created:', newUser);
};
createUser();
Reading Users
const getUsers = async () => {
const users = await User.findAll();
console.log('Users:', users);
};
getUsers();
Updating a User
const updateUser = async (id) => {
await User.update({ age: 35 }, { where: { id } });
console.log('User updated');
};
Deleting a User
const deleteUser = async (id) => {
await User.destroy({ where: { id } });
console.log('User deleted');
};
________________________________________
Conclusion
This guide covered:
• Setting up MongoDB with Mongoose and performing CRUD operations
• Setting up PostgreSQL with Sequelize and performing CRUD operations
Both MongoDB and PostgreSQL have their strengths. Choose MongoDB if you need a flexible schema and scalability, and PostgreSQL if you need relational integrity and structured queries.
Leave a Comment