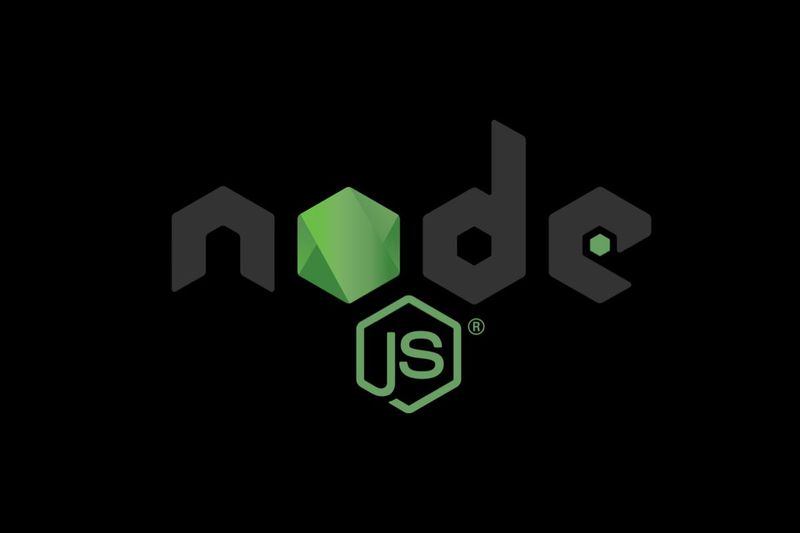
Introduction
Authentication and authorization are fundamental aspects of application security. Authentication verifies the identity of a user, while authorization determines the actions a user is allowed to perform.
This guide will cover:
- Handling user authentication
- JWT (JSON Web Tokens) authentication
- OAuth and third-party login (Google, Facebook)
- Role-based access control (RBAC)
1. Handling User Authentication
Authentication ensures that users are who they claim to be. Common authentication methods include:
a) Password-Based Authentication
- Users provide a username/email and password.
- Best practices:
- Store passwords securely using bcrypt or Argon2.
- Enforce strong password policies.
- Implement rate limiting to prevent brute-force attacks.
b) Multi-Factor Authentication (MFA)
- Adds an extra layer of security using:
- SMS/Email verification
- Authenticator apps (Google Authenticator, Authy)
- Biometric authentication (fingerprint, facial recognition)
c) Session-Based Authentication
- The server stores a session ID in cookies after successful login.
- Drawbacks:
- Not suitable for stateless applications.
- Requires session storage (database, Redis, etc.).
2. JWT (JSON Web Tokens) Authentication
JWT is a compact, self-contained token used for securely transmitting information.
a) Structure of a JWT
A JWT consists of three parts:
- Header: Specifies the algorithm (e.g., HS256).
- Payload: Contains user data (e.g., user ID, role).
- Signature: Ensures token integrity.
Example JWT:
{ "header": { "alg": "HS256", "typ": "JWT" }, "payload": { "user_id": 123, "role": "admin" }, "signature": "abc123xyz" }
b) How JWT Authentication Works
- User logs in with credentials.
- Server verifies credentials and generates a JWT.
- Client stores the JWT (local storage or cookies).
- JWT is sent in every request (
Authorization: Bearer <token>
). - Server validates the JWT and grants access.
c) Best Practices
- Use short-lived tokens (e.g., 15 minutes) and refresh tokens.
- Store tokens securely (use HTTPOnly cookies instead of local storage).
- Implement token revocation for security.
3. OAuth and Third-Party Login (Google, Facebook)
OAuth 2.0 is a protocol that allows third-party authentication without exposing user credentials.
a) OAuth Flow
- User clicks "Login with Google/Facebook".
- Redirected to provider's authorization server.
- User grants permission.
- Provider returns an authorization code.
- App exchanges the code for an access token.
- App uses token to authenticate API requests.
b) Implementing OAuth (Google Example)
- Register your app on Google Developer Console.
- Get Client ID and Client Secret.
- Use Google's OAuth endpoints for authentication.
Example OAuth URL:
https://accounts.google.com/o/oauth2/auth?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code&scope=email%20profile
- Exchange the authorization code for an access token.
- Fetch user data using the access token.
4. Role-Based Access Control (RBAC)
RBAC restricts access based on user roles (e.g., Admin, User, Moderator).
a) Defining Roles & Permissions
b) Implementing RBAC in Code (Node.js Example)
const roles = { admin: ['create', 'read', 'update', 'delete'], editor: ['read', 'update'], user: ['read'] }; function checkPermission(role, action) { return roles[role]?.includes(action); } console.log(checkPermission('admin', 'delete')); // true console.log(checkPermission('user', 'delete')); // false
c) Middleware for Role-Based Access (Express.js)
function authorizeRole(role) { return (req, res, next) => { if (req.user.role !== role) { return res.status(403).json({ message: 'Access Denied' }); } next(); }; } app.get('/admin', authorizeRole('admin'), (req, res) => { res.send('Welcome Admin'); });
Conclusion
Authentication and authorization are essential for securing applications. This guide covered:
- User authentication (passwords, MFA, sessions)
- JWT authentication for stateless authentication
- OAuth & third-party login for seamless authentication
- Role-Based Access Control (RBAC) for managing permissions
Implement these best practices to build secure applications and protect user data.
Leave a Comment