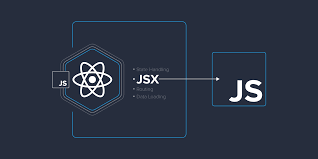
JSX (JavaScript XML) is a syntax extension for JavaScript, commonly used in React to describe the UI structure. It allows developers to write HTML-like code within JavaScript, making it easier to define components and manage UI elements.
One of the key features of JSX is its ability to include JavaScript expressions within curly braces {}
. This enables dynamic content rendering, conditional logic, and function execution inside JSX. In this blog, we will explore how to use JavaScript expressions in JSX effectively.
1. Inline Expressions in JSX
JSX allows us to embed JavaScript expressions directly within the markup using curly braces {}
. These expressions can include variables, mathematical operations, function calls, and more.
Example:
const name = "Obafemi"; const age = 30; function Greeting() { return ( <div> <h1>Hello, {name}!</h1> <p>Your age is {age + 1}.</p> </div> ); }
In the above example:
{name}
dynamically inserts the value of thename
variable.{age + 1}
performs a calculation and renders the result.
2. Conditional Rendering in JSX
Conditional rendering allows components to display different UI elements based on specific conditions. JSX supports conditional rendering using:
if
statements (used outside JSX)- The ternary operator (
condition ? trueExpression : falseExpression
) - The logical
&&
operator (condition && expression
)
Using if
(Outside JSX)
If statements cannot be used directly inside JSX, but we can use them before the return statement:
function UserGreeting({ isLoggedIn }) { let message; if (isLoggedIn) { message = "Welcome back!"; } else { message = "Please log in."; } return <h2>{message}</h2>; }
Using the Ternary Operator
The ternary operator is commonly used inside JSX for concise conditional rendering:
function UserGreeting({ isLoggedIn }) { return ( <h2>{isLoggedIn ? "Welcome back!" : "Please log in."}</h2> ); }
Using Logical &&
Operator
When rendering content conditionally, the &&
operator can be used to render elements based on a truthy condition:
function Notification({ hasNewMessages }) { return ( <div> <h1>Dashboard</h1> {hasNewMessages && <p>You have new messages!</p>} </div> ); }
- If
hasNewMessages
istrue
, the<p>
tag will be rendered. - If
hasNewMessages
isfalse
, nothing is displayed.
3. Using JavaScript Functions inside JSX
JSX allows calling JavaScript functions inside curly braces. This is useful when you need dynamic content generation or complex calculations.
Example:
function formatDate(date) { return date.toLocaleDateString(); } function DisplayDate() { return <p>Today's date is {formatDate(new Date())}.</p>; }
In this example:
- The
formatDate
function formats the current date. - It is called inside JSX using
{formatDate(new Date())}
.
Conclusion
JSX is a powerful feature in React that allows seamless integration of JavaScript expressions within UI components. By using inline expressions, conditional rendering techniques, and functions inside JSX, developers can create highly dynamic and interactive applications.
Understanding these concepts will help you write cleaner, more maintainable React code. Keep experimenting with JSX, and you will master React development in no time!
Leave a Comment