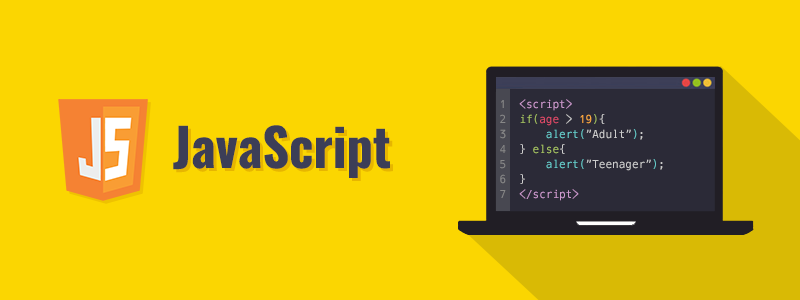
JavaScript modules are a crucial part of modern web development, allowing developers to structure and manage code efficiently. Modules help in organizing code, reducing global scope pollution, and improving maintainability. In this blog, we will explore JavaScript modules in-depth, covering:
- Export and Import in ES Modules
- CommonJS vs ES Modules
- Dynamic Imports
1. Export and Import in ES Modules
What Are ES Modules?
ES Modules (ECMAScript Modules) were introduced in ES6 (ECMAScript 2015) to provide a standardized way of organizing code into reusable pieces. Unlike traditional script loading, ES modules allow for better dependency management and code separation.
Exporting in ES Modules
You can export functions, objects, or primitive values from one module so they can be used in another.
Named Exports
Named exports allow you to export multiple values from a module.
// math.js export const add = (a, b) => a + b; export const subtract = (a, b) => a - b;
To import named exports:
// main.js import { add, subtract } from './math.js'; console.log(add(5, 3)); // 8 console.log(subtract(5, 3)); // 2
Default Exports
A module can have only one default export.
// greeting.js export default function greet(name) { return `Hello, ${name}!`; }
To import a default export:
// main.js import greet from './greeting.js'; console.log(greet('John')); // Hello, John!
2. CommonJS vs ES Modules
JavaScript has historically used different module systems. The most common two are CommonJS and ES Modules.
CommonJS (CJS)
CommonJS is the module system used in Node.js before ES Modules were introduced.
How CommonJS Works
- Uses
require()
for importing modules. - Uses
module.exports
orexports
to expose functionalities. - Synchronous loading (not suitable for browsers without bundling tools like Webpack).
Example:
// math.js (CommonJS) module.exports = { add: (a, b) => a + b, subtract: (a, b) => a - b, };
Importing in another file:
// main.js const math = require('./math'); console.log(math.add(4, 2)); // 6
ES Modules (ESM)
- Uses
import
andexport
syntax. - Supports asynchronous loading.
- Works natively in modern browsers and can be used in Node.js (with
type: "module"
inpackage.json
).
Key Differences
3. Dynamic Imports
Dynamic imports allow you to load modules conditionally or asynchronously, which is useful for code-splitting and performance optimization.
Using import()
Unlike static imports, import()
returns a Promise, enabling asynchronous loading.
Example:
// main.js async function loadModule() { const { add } = await import('./math.js'); console.log(add(2, 3)); // 5 } loadModule();
Steps to Use Import and Export in a Website
- Create a JavaScript module (
math.js
) - This file will contain the exported functions.
javascript CopyEdit // math.js export function add(a, b) { return a + b; } export function subtract(a, b) { return a - b; }
- Create the main JavaScript file (
main.js
) - Import the functions and use them.
javascript CopyEdit // main.js import { add, subtract } from './math.js'; console.log(add(5, 3)); // 8 console.log(subtract(5, 3)); // 2 // Display result in HTML document.getElementById("result").textContent = `5 + 3 = ${add(5, 3)}`;
- Create an HTML file (
index.html
) - Load
main.js
as a module.
html CopyEdit <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Modules Example</title> </head> <body> <h1>JavaScript Modules Example</h1> <p id="result"></p> <script type="module" src="main.js"></script> </body> </html>
Key Points
- Use
type="module"
in the<script>
tag when importing modules. - Use
export
in the module file (math.js
). - Use
import
in the main JavaScript file (main.js
). - Ensure files are served via an HTTP server, as some browsers block module imports from local file URLs (
file://
).
Benefits of Dynamic Imports
- Load modules on demand, reducing initial bundle size.
- Useful for lazy loading components in frameworks like React.
- Helps with performance optimizations in web applications.
Conclusion
JavaScript modules provide an effective way to structure and manage code. Understanding the differences between CommonJS and ES Modules is crucial for modern development. Additionally, dynamic imports offer flexibility and performance improvements. With ES Modules now natively supported in browsers and Node.js, adopting them ensures a more maintainable and scalable codebase.
By mastering JavaScript modules, you can write cleaner, more efficient, and modular code that is easier to maintain and extend.
Leave a Comment