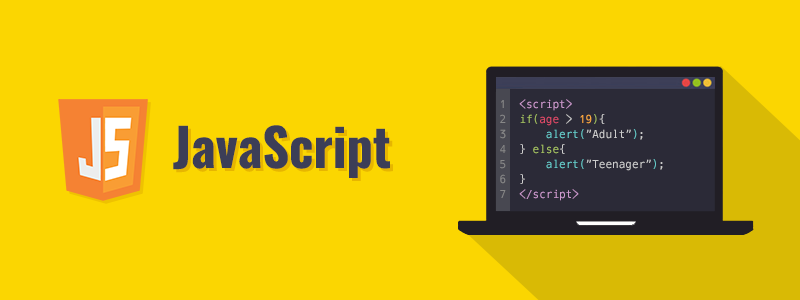
When working with JavaScript, one of the fundamental tasks is selecting elements from the DOM (Document Object Model). JavaScript provides several methods to achieve this, each serving different purposes and offering varying levels of flexibility. In this guide, we'll explore all the available methods for selecting DOM elements.
1. Selecting by ID: document.getElementById()
The getElementById
method allows you to select an element by its unique ID.
const element = document.getElementById("myElement"); console.log(element);
Characteristics:
- Returns a single
HTMLElement
. - If no element matches, it returns
null
. - Fastest method for element selection.
2. Selecting by Class Name: document.getElementsByClassName()
The getElementsByClassName
method selects all elements that share a specific class name.
const elements = document.getElementsByClassName("myClass"); console.log(elements);
Characteristics:
- Returns an HTMLCollection (live collection that updates when the DOM changes).
- Can be accessed like an array but lacks array methods.
3. Selecting by Tag Name: document.getElementsByTagName()
This method selects elements based on their tag name (e.g., div
, p
, span
).
const elements = document.getElementsByTagName("div"); console.log(elements);
Characteristics:
- Returns an HTMLCollection.
- Useful for selecting all elements of a certain type.
4. Selecting with CSS Selectors: document.querySelector()
The querySelector
method allows selecting the first element that matches a given CSS selector.
const element = document.querySelector(".myClass"); console.log(element);
Characteristics:
- Returns the first matching element or
null
if none is found. - Supports complex CSS selectors.
5. Selecting Multiple Elements with CSS Selectors: document.querySelectorAll()
The querySelectorAll
method selects all elements that match a given CSS selector.
const elements = document.querySelectorAll(".myClass"); console.log(elements);
Characteristics:
- Returns a NodeList (static collection that does not update dynamically).
- Can be iterated using
forEach
. - Supports CSS selectors.
6. Selecting Elements from a Specific Element
Instead of selecting from document
, you can use these methods on specific elements:
const container = document.getElementById("container"); const items = container.getElementsByClassName("item"); console.log(items);
Works With:
getElementsByClassName()
getElementsByTagName()
querySelector()
querySelectorAll()
7. Selecting the Active Element: document.activeElement
This property returns the element currently focused in the document.
const activeElement = document.activeElement; console.log(activeElement);
Use Case:
- Useful for tracking user interactions, such as input field focus.
8. Selecting Elements in Forms
document.forms
Retrieves all forms in the document.
const forms = document.forms; console.log(forms);
document.forms["myForm"]
Access a specific form by name or index.
const myForm = document.forms["myForm"]; console.log(myForm);
form.elements
Retrieve all input elements inside a form.
const inputs = myForm.elements; console.log(inputs);
9. Selecting Elements by Name: document.getElementsByName()
The getElementsByName
method selects elements based on the name
attribute.
const elements = document.getElementsByName("username"); console.log(elements);
Characteristics:
- Returns a NodeList.
- Mainly used for radio buttons and form inputs.
10. Selecting the Root Element: document.documentElement
The documentElement
property selects the root <html>
element.
const root = document.documentElement; console.log(root);
Use Case:
- Used for manipulating the entire document (e.g., scrolling effects).
11. Selecting the Body Element: document.body
The body
property selects the <body>
element.
const body = document.body; console.log(body);
Use Case:
- Useful for dynamically modifying page content.
12. Selecting the Head Element: document.head
The head
property selects the <head>
element.
const head = document.head; console.log(head);
Use Case:
- Useful for modifying meta tags dynamically.
Conclusion
Understanding these selection methods is crucial for efficient DOM manipulation. Choosing the right method depends on your needs:
- For unique elements:
getElementById()
- For multiple elements:
getElementsByClassName()
,getElementsByTagName()
,querySelectorAll()
- For flexibility:
querySelector()
andquerySelectorAll()
- For form handling:
getElementsByName()
,document.forms
By mastering these techniques, you'll be able to efficiently navigate and manipulate the DOM in your JavaScript projects.
Leave a Comment