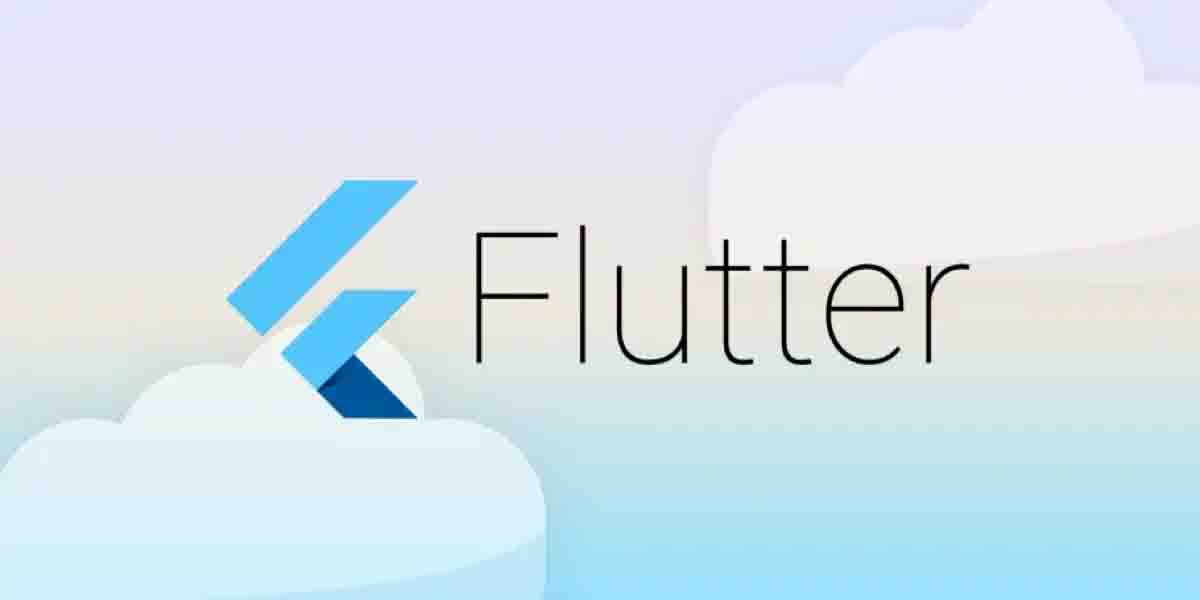
Managing data efficiently is a crucial aspect of mobile app development. Flutter provides multiple options for local storage and database management, each with its unique advantages. In this guide, we will explore various storage solutions available in Flutter:
- Shared Preferences (Key-Value Storage)
- SQLite Database with sqflite
- Hive - NoSQL Database
- Object Relational Mapping (ORM) in Flutter
- Firebase Firestore Database
1. Shared Preferences (Key-Value Storage)
Shared Preferences is a simple key-value storage solution for storing small amounts of data persistently, such as user settings and app preferences.
When to Use:
- Storing user preferences (e.g., dark mode, language settings)
- Saving authentication tokens or session data
- Caching lightweight data
Installation:
dependencies: shared_preferences: ^2.2.0
Implementation:
import 'package:shared_preferences/shared_preferences.dart'; Future<void> saveData(String key, String value) async { final prefs = await SharedPreferences.getInstance(); await prefs.setString(key, value); } Future<String?> getData(String key) async { final prefs = await SharedPreferences.getInstance(); return prefs.getString(key); }
Pros:
- Lightweight and fast
- Easy to use
- Good for storing small data
Cons:
- Not suitable for complex data structures
- Limited storage capacity
2. SQLite Database with sqflite
SQLite is a powerful relational database that allows structured data storage.
When to Use:
- Storing structured data with relationships
- Managing offline-first applications
- Handling complex queries
Installation:
dependencies: sqflite: ^2.3.0 path_provider: ^2.0.15
Implementation:
import 'package:sqflite/sqflite.dart'; import 'package:path/path.dart'; Future<Database> initDatabase() async { return openDatabase( join(await getDatabasesPath(), 'app_database.db'), onCreate: (db, version) { return db.execute('CREATE TABLE users(id INTEGER PRIMARY KEY, name TEXT)'); }, version: 1, ); } Future<void> insertUser(Database db, Map<String, dynamic> user) async { await db.insert('users', user, conflictAlgorithm: ConflictAlgorithm.replace); }
Pros:
- Structured data storage
- SQL query support
- Persistent data storage
Cons:
- Requires schema design
- Can be complex to manage relationships
3. Hive - NoSQL Database
Hive is a lightweight and fast NoSQL database for Flutter apps.
When to Use:
- Storing local data in key-value pairs
- Handling offline data in apps
- Faster performance than SQLite for simple queries
Installation:
dependencies: hive: ^2.2.3 hive_flutter: ^1.1.0
Implementation:
import 'package:hive/hive.dart'; import 'package:hive_flutter/hive_flutter.dart'; Future<void> initHive() async { await Hive.initFlutter(); await Hive.openBox('settings'); } Future<void> saveSetting(String key, String value) async { var box = Hive.box('settings'); box.put(key, value); } Future<String?> getSetting(String key) async { var box = Hive.box('settings'); return box.get(key); }
Pros:
- High-speed operations
- No need for structured schema
- Offline storage
Cons:
- Not suitable for relational data
4. Object Relational Mapping (ORM) in Flutter
ORM allows developers to interact with databases using Dart objects instead of SQL queries.
Popular Flutter ORM Packages:
- Drift (formerly moor)
- Floor
Example Using Drift:
dependencies: drift: ^2.9.0 sqlite3_flutter_libs: ^0.5.0 import 'package:drift/drift.dart'; import 'package:drift/native.dart'; part 'database.g.dart'; @DataClassName("User") class Users extends Table { IntColumn get id => integer().autoIncrement()(); TextColumn get name => text()(); } @DriftDatabase(tables: [Users]) class AppDatabase extends _$AppDatabase { AppDatabase() : super(NativeDatabase.memory()); }
Pros:
- Simplifies database interactions
- Reduces boilerplate code
- Strongly typed queries
Cons:
- Additional learning curve
- Slightly larger app size
5. Firebase Firestore Database
Firebase Firestore is a cloud-hosted NoSQL database for scalable real-time applications.
When to Use:
- Real-time data sync across devices
- Scalable applications
- Cloud-based storage
Installation:
dependencies: cloud_firestore: ^4.9.3 firebase_core: ^2.15.0
Implementation:
import 'package:cloud_firestore/cloud_firestore.dart'; Future<void> addUser(String name) async { await FirebaseFirestore.instance.collection('users').add({'name': name}); } Stream<QuerySnapshot> getUsers() { return FirebaseFirestore.instance.collection('users').snapshots(); }
Pros:
- Real-time syncing
- Scalable and secure
- Serverless architecture
Cons:
- Requires internet connection
- Costs for large-scale applications
Leave a Comment