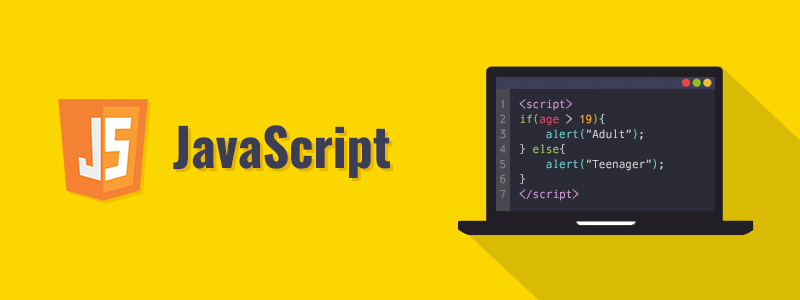
When writing JavaScript code, errors are inevitable. Whether they come from user input, network failures, or logical mistakes, handling them properly ensures a smooth user experience and prevents application crashes. In this guide, we'll explore JavaScript's error handling mechanisms and debugging techniques, focusing on:
try...catch
finally
blockthrow
keyword- Debugging with Browser DevTools
1. The try...catch
Statement
The try...catch
statement allows you to handle exceptions (errors) gracefully instead of stopping script execution.
Syntax:
try { // Code that may throw an error } catch (error) { // Code to handle the error }
Example:
try { let result = 10 / 0; // No error, but division by zero returns Infinity console.log(result); let user = JSON.parse('{ invalid JSON }'); // This will throw an error } catch (error) { console.error("An error occurred:", error.message); }
In the above example:
- The first operation (
10 / 0
) doesn’t throw an error but results inInfinity
. - The
JSON.parse()
function throws an error due to invalid JSON syntax. - The
catch
block catches and logs the error, preventing the script from crashing.
2. The finally
Block
The finally
block runs regardless of whether an error occurs or not. It is typically used for cleanup operations, such as closing connections or freeing up resources.
Syntax:
try { // Code that may throw an error } catch (error) { // Code to handle the error } finally { // Code that runs no matter what }
Example:
function fetchData() { try { console.log("Fetching data..."); throw new Error("Network failure"); } catch (error) { console.error("Error:", error.message); } finally { console.log("Operation completed."); } } fetchData();
Output:
Fetching data... Error: Network failure Operation completed.
The finally
block executes even if an error occurs or if the try block runs successfully.
3. The throw
Keyword
The throw
keyword allows you to manually trigger an error.
Syntax:
throw new Error("Custom error message");
Example:
function divide(a, b) { if (b === 0) { throw new Error("Cannot divide by zero"); } return a / b; } try { console.log(divide(10, 2)); // Works fine console.log(divide(10, 0)); // Throws an error } catch (error) { console.error("Caught an error:", error.message); }
4. Debugging with Browser DevTools
JavaScript provides powerful debugging tools via Browser Developer Tools (DevTools). You can use them to inspect variables, pause execution, and analyze performance.
4.1. Console Logging (console.log
)
A simple way to debug is by logging output to the console.
let x = 5; console.log("The value of x is:", x);
Shortcut: Open DevTools with F12
or Ctrl + Shift + I
(Windows/Linux) or Cmd + Option + I
(Mac).
4.2. Using debugger
The debugger
keyword pauses script execution at a specific line.
function test() { let a = 10; debugger; // Execution pauses here let b = 20; console.log(a + b); } test();
Once paused, you can inspect variables and step through the code in DevTools.
4.3. Setting Breakpoints
- Open DevTools (
F12
orCtrl + Shift + I
). - Navigate to the Sources tab.
- Locate your JavaScript file.
- Click on a line number to set a breakpoint.
- Reload the page and execution will pause at the breakpoint.
4.4. Watching Variables
You can monitor variable values in DevTools:
- Open the Sources tab.
- In the right-hand panel, add variables to the Watch section.
4.5. Checking Network Requests
Use the Network tab in DevTools to inspect AJAX requests:
- Open DevTools and go to the Network tab.
- Reload the page.
- Click on a request to see its details (headers, response, timing, etc.).
Conclusion
Effective error handling and debugging improve application stability and performance. JavaScript provides try...catch
, finally
, and throw
for handling errors, while DevTools helps diagnose and fix issues efficiently.
By mastering these techniques, you can write robust and bug-free JavaScript applications!
Leave a Comment