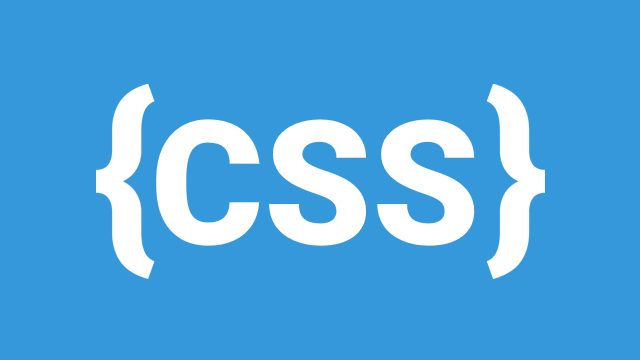
The CSS Box Model is a fundamental concept that defines how elements are structured and displayed on a web page. Every HTML element is represented as a rectangular box that consists of four main parts:
- Content - The actual text, image, or other elements inside the box.
- Padding - Space between the content and the border.
- Border - A surrounding boundary around the padding and content.
- Margin - Space between the element and adjacent elements.
This model helps in designing layouts by controlling spacing and element behavior.
1. Components of the Box Model
Each element’s total size is determined by adding the values of the above components together:
Total Width=Content Width+Padding (Left + Right)+Border (Left + Right)+Margin (Left + Right)\text{Total Width} = \text{Content Width} + \text{Padding (Left + Right)} + \text{Border (Left + Right)} + \text{Margin (Left + Right)}Total Width=Content Width+Padding (Left + Right)+Border (Left + Right)+Margin (Left + Right)Total Height=Content Height+Padding (Top + Bottom)+Border (Top + Bottom)+Margin (Top + Bottom)\text{Total Height} = \text{Content Height} + \text{Padding (Top + Bottom)} + \text{Border (Top + Bottom)} + \text{Margin (Top + Bottom)}Total Height=Content Height+Padding (Top + Bottom)+Border (Top + Bottom)+Margin (Top + Bottom)
Now, let’s break down each component:
a) Content
The content is the area where text, images, or other HTML elements appear.
Example:
css CopyEdit .box { width: 200px; height: 100px; background-color: lightblue; }
This defines an element with a width of 200px and a height of 100px.
b) Padding
Padding is the space inside the element, between the content and the border. It increases the visual size of the element without affecting surrounding elements.
Example:
css CopyEdit .box { padding: 20px; }
This adds 20px of space inside the box between the content and the border.
You can also set different padding values for each side:
css CopyEdit .box { padding-top: 10px; padding-right: 15px; padding-bottom: 10px; padding-left: 15px; }
Or shorthand:
css CopyEdit .box { padding: 10px 15px; /* Top & Bottom: 10px, Left & Right: 15px */ }
c) Border
The border surrounds the padding and content and can have different styles, widths, and colors.
Example:
css CopyEdit .box { border: 3px solid black; }
This applies a 3px solid black border around the element.
Other border styles:
css CopyEdit border: 2px dashed red; /* Dashed border */ border: 2px dotted blue; /* Dotted border */ border: 2px double green; /* Double border */
d) Margin
Margin is the space outside the element’s border. It affects the distance between this element and surrounding elements.
Example:
css CopyEdit .box { margin: 20px; }
This creates 20px of space around all sides of the element.
You can set different margins for each side:
css CopyEdit .box { margin-top: 10px; margin-right: 15px; margin-bottom: 10px; margin-left: 15px; }
Or shorthand:
css CopyEdit .box { margin: 10px 15px; /* Top & Bottom: 10px, Left & Right: 15px */ }
2. Width and Height in the Box Model
By default, width and height apply only to the content area, excluding padding, border, and margin.
Example:
css CopyEdit .box { width: 200px; height: 100px; padding: 20px; border: 5px solid black; }
The total width and height of this box will be:
Total Width=200px+(20px×2)+(5px×2)=250px\text{Total Width} = 200px + (20px \times 2) + (5px \times 2) = 250pxTotal Width=200px+(20px×2)+(5px×2)=250pxTotal Height=100px+(20px×2)+(5px×2)=150px\text{Total Height} = 100px + (20px \times 2) + (5px \times 2) = 150pxTotal Height=100px+(20px×2)+(5px×2)=150px
To make width and height include padding and border, use:
css CopyEdit .box { box-sizing: border-box; }
Now, the declared width and height include padding and border, preventing layout issues.
3. Overflow Handling
When content inside an element is larger than the box’s defined width or height, it may overflow. The overflow
property controls how this overflow is handled.
a) Overflow Values
visible
(default) → Content overflows outside the box.hidden
→ Hides overflowing content.scroll
→ Adds a scrollbar to view hidden content.auto
→ Adds a scrollbar only when needed.
Example:
css CopyEdit .box { width: 200px; height: 100px; overflow: hidden; }
This hides any content that extends beyond 200px width and 100px height.
To allow scrolling:
css CopyEdit .box { overflow: scroll; }
To add scrollbars only when needed:
css CopyEdit .box { overflow: auto; }
To control horizontal and vertical overflow separately:
css CopyEdit .box { overflow-x: scroll; /* Horizontal scrolling */ overflow-y: hidden; /* No vertical scrolling */ }
Conclusion
The CSS Box Model is crucial for understanding how elements interact with one another on a web page. It determines spacing, sizing, and layout. By mastering the box model, width/height, and overflow handling, you can create visually appealing and well-structured web designs.
Leave a Comment