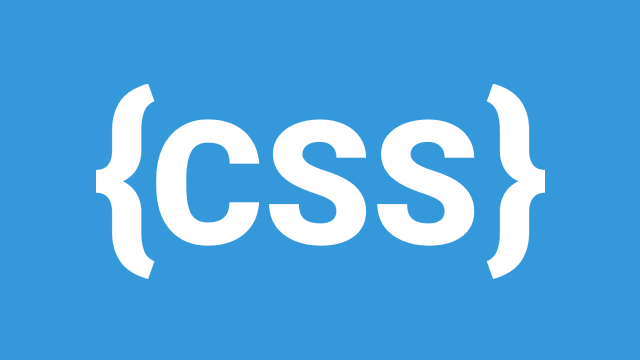
CSS is an essential part of web development, responsible for the visual presentation of a website. However, writing maintainable, scalable, and performant CSS requires following best practices. This guide will cover key aspects such as writing maintainable CSS, using preprocessors like SASS and LESS, optimizing performance, and ensuring browser compatibility.
Writing Maintainable and Scalable CSS
Maintainability and scalability in CSS mean writing styles that are easy to understand, modify, and extend without breaking existing designs. Here are best practices to achieve this:
1. Follow a Naming Convention (BEM, SMACSS, OOCSS)
- BEM (Block Element Modifier): A popular methodology that makes CSS easier to understand and scale.
.button {} .button--primary {} .button__icon {}
- SMACSS (Scalable and Modular Architecture for CSS): Focuses on categorizing CSS into different styles such as base, layout, module, state, and theme.
- OOCSS (Object-Oriented CSS): Encourages code reuse and separation of structure from skin.
2. Use Modular CSS
- Break styles into small, reusable components.
- Keep styles encapsulated to avoid global conflicts.
3. Avoid Deep Nesting
- Keep CSS selectors shallow to improve readability and performance.
/* Avoid this */ .header .nav .menu .menu-item a {} /* Prefer this */ .menu-item a {}
4. Keep Specificity Low
- Use class selectors instead of IDs for styling.
- Avoid
!important
unless absolutely necessary.
5. Use Variables and Mixins
- Define reusable values for colours, fonts, and spacing.
:root { --primary-color: #3498db; --font-size-lg: 18px; }
CSS Preprocessors (SASS, LESS)
CSS preprocessors like SASS and LESS extend CSS by adding features such as variables, mixins, functions, and nesting.
1. SASS (Syntactically Awesome Stylesheets)
- Allows variables, nesting, mixins, and functions.
- Supports .scss and .sass syntax.
- Example:
$primary-color: #3498db; .button { background: $primary-color; &:hover { background: darken($primary-color, 10%); } }
2. LESS (Leaner Style Sheets)
- Similar to SASS but uses
.less
syntax. - Example:
@primary-color: #3498db; .button { background: @primary-color; &:hover { background: darken(@primary-color, 10%); } }
Benefits of Preprocessors
- Maintainability: Reduces code duplication.
- Scalability: Encourages modular and structured styling.
- Reusability: Use mixins and functions to reduce repetitive code.
Optimizing CSS for Performance
1. Minify and Compress CSS
- Use tools like CSSNano, UglifyCSS, or PostCSS to reduce file size.
- Example command using CSSNano:
cssnano styles.css styles.min.css
2. Use Critical CSS
- Load essential CSS first to improve page rendering speed.
- Example with critical CSS in
<head>
:
<style> body { font-family: Arial, sans-serif; background: #fff; } </style>
- Defer loading of non-critical styles with
media="print"
:
<link rel="stylesheet" href="styles.css" media="print" onload="this.media='all'">
3. Reduce Unused CSS
- Use tools like PurgeCSS to remove unused styles.
- Example:
purgecss --css styles.css --content index.html
4. Use CSS Grid and Flexbox Instead of Floats
- Modern layout techniques reduce unnecessary styles and improve responsiveness.
.container { display: flex; justify-content: space-between; }
5. Optimize Fonts and Icons
- Use font-display to load fonts asynchronously:
@font-face { font-family: 'CustomFont'; src: url('font.woff2') format('woff2'); font-display: swap; }
- Prefer SVG over font icons for better performance.
Browser Compatibility and Vendor Prefixes
Ensuring your CSS works across different browsers is critical for a smooth user experience.
1. Use Autoprefixer
- Automates vendor prefixing for better compatibility.
- Example:
npx postcss styles.css --use autoprefixer -o styles-prefixed.css
2. Use Feature Detection with Modernizr
- Detects browser capabilities and applies appropriate styles.
- Example:
if (Modernizr.flexbox) { document.body.classList.add("flexbox-enabled"); }
3. Provide Fallbacks for Older Browsers
- Example of CSS Grid fallback:
.container { display: flex; } @supports (display: grid) { .container { display: grid; grid-template-columns: repeat(3, 1fr); } }
4. Test Across Multiple Browsers
- Use tools like BrowserStack, CrossBrowserTesting, or Can I Use to check compatibility.
Conclusion
By following these CSS best practices, developers can create maintainable, scalable, and performant styles. Preprocessors like SASS and LESS enhance code organization, while optimization techniques improve loading speeds. Ensuring browser compatibility ensures a seamless user experience across different devices and browsers. Implement these strategies to build high-quality, future-proof stylesheets for your projects.
Leave a Comment