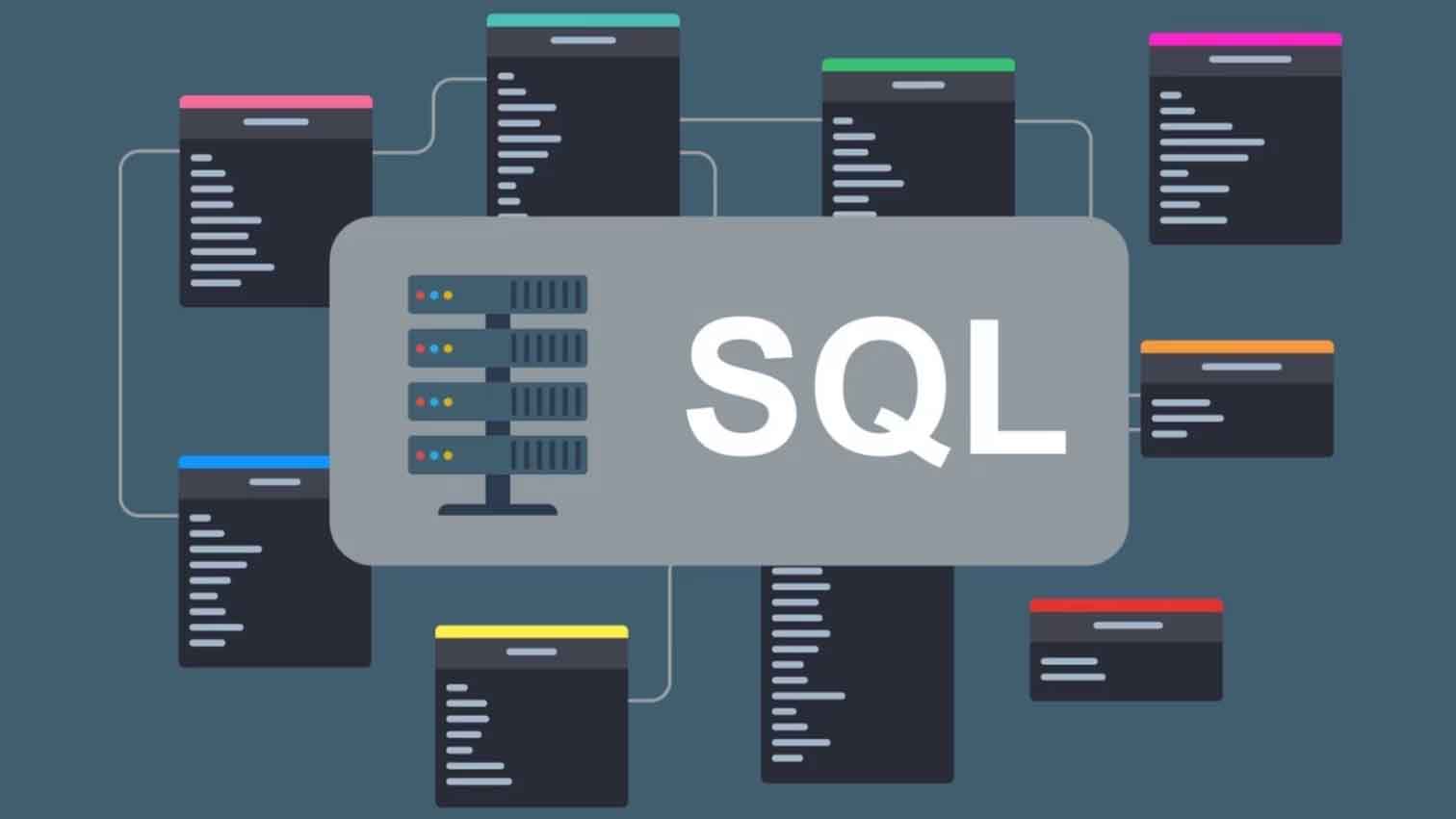
SQL (Structured Query Language) is a powerful tool for managing and querying relational databases. While basic SQL covers SELECT, INSERT, UPDATE, and DELETE statements, mastering advanced features allows developers to optimize performance, simplify complex queries, and handle sophisticated data structures. In this blog, we will explore some of the most powerful advanced SQL features:
- Common Table Expressions (CTEs)
- Window Functions (ROW_NUMBER, RANK, DENSE_RANK)
- Recursive Queries
- JSON Support in SQL
- Full-Text Search
Let's dive into each of these features with explanations and examples.
1. Common Table Expressions (CTEs)
CTEs provide a way to write cleaner and more readable queries by allowing you to define temporary result sets that can be referenced within the main query. They improve query structure and are particularly useful for recursive queries and breaking down complex SQL logic.
Syntax:
WITH cte_name AS ( SELECT column1, column2 FROM table_name WHERE condition ) SELECT * FROM cte_name;
Example:
Consider a scenario where you want to get the employees with a salary above the department average.
WITH DepartmentAverage AS ( SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id ) SELECT e.employee_name, e.salary, e.department_id FROM employees e JOIN DepartmentAverage da ON e.department_id = da.department_id WHERE e.salary > da.avg_salary;
CTEs improve query readability and can be reused multiple times within the main query.
2. Window Functions (ROW_NUMBER, RANK, DENSE_RANK)
Window functions allow calculations across a subset of rows related to the current row without collapsing the result set.
ROW_NUMBER, RANK, and DENSE_RANK:
- ROW_NUMBER() assigns a unique number to each row within a partition.
- RANK() assigns ranks, but skips numbers for duplicates.
- DENSE_RANK() assigns ranks without gaps for duplicates.
Example:
SELECT employee_name, department_id, salary, ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary DESC) AS row_num, RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) AS rank_num, DENSE_RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) AS dense_rank_num FROM employees;
This query ranks employees within each department based on salary.
3. Recursive Queries
Recursive queries are useful for hierarchical data, such as organizational structures and category trees.
Syntax:
WITH RECURSIVE cte_name AS ( SELECT column1, column2 FROM table WHERE condition -- Base case UNION ALL SELECT column1, column2 FROM table JOIN cte_name ON condition -- Recursive case ) SELECT * FROM cte_name;
Example:
Retrieving an employee hierarchy:
WITH RECURSIVE EmployeeHierarchy AS ( SELECT employee_id, employee_name, manager_id, 1 AS level FROM employees WHERE manager_id IS NULL -- Start from top-level manager UNION ALL SELECT e.employee_id, e.employee_name, e.manager_id, eh.level + 1 FROM employees e JOIN EmployeeHierarchy eh ON e.manager_id = eh.employee_id ) SELECT * FROM EmployeeHierarchy;
This query builds an employee hierarchy from the CEO to the lowest-level employees.
4. JSON Support in SQL
Modern SQL databases support JSON data types, allowing structured data to be stored and queried efficiently.
Example: Storing and Querying JSON Data
CREATE TABLE products ( id SERIAL PRIMARY KEY, name VARCHAR(255), details JSONB ); INSERT INTO products (name, details) VALUES ('Laptop', '{"brand": "Dell", "specs": {"ram": "16GB", "storage": "512GB SSD"}}'); SELECT name, details->'brand' AS brand FROM products;
This query extracts the brand field from the JSON data stored in the details
column.
5. Full-Text Search
Full-text search allows efficient text searching and ranking in SQL databases, making it ideal for applications with search functionalities.
Example: Full-Text Search in PostgreSQL
ALTER TABLE articles ADD COLUMN search_vector tsvector; UPDATE articles SET search_vector = to_tsvector('english', content); SELECT title FROM articles WHERE search_vector @@ to_tsquery('database & performance');
This query searches for articles related to "database" and "performance" using full-text indexing.
Conclusion
Mastering advanced SQL features such as CTEs, window functions, recursive queries, JSON support, and full-text search enables developers to write more efficient and powerful queries. These techniques enhance database performance and simplify complex data retrieval tasks.
By integrating these features into your SQL toolkit, you can unlock the full potential of relational databases and improve data-driven applications.
Leave a Comment