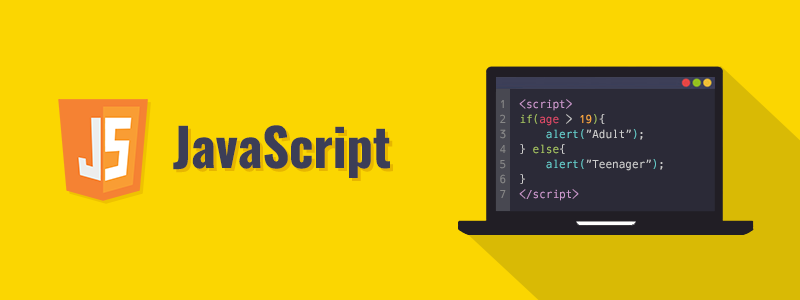
JavaScript has evolved beyond simple scripting, enabling developers to build complex, high-performance applications. In this blog, we explore five advanced JavaScript topics that every developer should understand: WebSockets, Service Workers, WebAssembly, JavaScript Memory Management, and Performance Optimization Techniques.
1. WebSockets: Real-time Communication in JavaScript
What Are WebSockets?
WebSockets provide a full-duplex communication channel over a single TCP connection. Unlike HTTP, which follows a request-response model, WebSockets allow bidirectional, real-time communication between a client and a server.
How Do WebSockets Work?
- The client sends a WebSocket handshake request to the server.
- The server responds, and a persistent connection is established.
- Both client and server can send messages asynchronously.
Example Implementation
const socket = new WebSocket('wss://example.com/socket'); socket.onopen = function(event) { console.log('Connected to WebSocket server'); socket.send('Hello, Server!'); }; socket.onmessage = function(event) { console.log('Message from server:', event.data); }; socket.onclose = function(event) { console.log('Disconnected'); };
Use Cases
- Live chat applications
- Real-time stock price updates
- Multiplayer gaming
- IoT applications
2. Service Workers: Enabling Offline Functionality
What Are Service Workers?
A Service Worker is a script that runs in the background, separate from the main browser thread. It enables features like caching, background sync, and push notifications.
How Service Workers Work
- The service worker is registered in the browser.
- It intercepts network requests and serves cached responses when offline.
- It updates itself in the background.
Example Implementation
if ('serviceWorker' in navigator) { navigator.serviceWorker.register('/service-worker.js') .then(reg => console.log('Service Worker Registered', reg)) .catch(err => console.error('Service Worker Registration Failed', err)); }
Use Cases
- Progressive Web Apps (PWAs)
- Offline browsing
- Background sync and push notifications
3. WebAssembly: Running High-Performance Code in the Browser
What Is WebAssembly (Wasm)?
WebAssembly is a binary instruction format that allows running compiled languages (C, C++, Rust) in the browser at near-native speed.
How WebAssembly Works
- Code is written in a language like C++ or Rust.
- It is compiled to WebAssembly (.wasm file).
- JavaScript loads and executes the WebAssembly module.
Example: Running a WebAssembly Module
fetch('module.wasm') .then(response => response.arrayBuffer()) .then(bytes => WebAssembly.instantiate(bytes)) .then(results => { console.log('WebAssembly Loaded', results); });
Use Cases
- High-performance web applications
- Game engines
- Image and video processing
4. JavaScript Memory Management
Understanding Memory Allocation in JavaScript
JavaScript manages memory using:
- Allocation: Variables and objects are stored in memory.
- Garbage Collection: The JavaScript engine automatically removes unused memory using algorithms like Mark-and-Sweep.
Avoiding Memory Leaks
Common causes of memory leaks:
- Unused event listeners
- Global variables
- Circular references
Best Practices for Memory Optimization
- Use
WeakMap
andWeakSet
for objects with dynamic lifetimes. - Avoid excessive DOM elements.
- Clear event listeners when not needed.
5. Performance Optimization Techniques
Key Performance Bottlenecks
- Unoptimized loops and recursion
- Excessive DOM manipulation
- Heavy computations on the main thread
Techniques to Optimize Performance
1. Debouncing and Throttling
function debounce(func, delay) { let timeout; return function(...args) { clearTimeout(timeout); timeout = setTimeout(() => func.apply(this, args), delay); }; }
Use this for optimizing input events.
2. Minimizing Repaints and Reflows
Batch DOM updates:
const fragment = document.createDocumentFragment(); for (let i = 0; i < 1000; i++) { const div = document.createElement('div'); div.textContent = `Item ${i}`; fragment.appendChild(div); } document.body.appendChild(fragment);
3. Lazy Loading Images and Content
<img src="placeholder.jpg" data-src="real-image.jpg" class="lazy-load">
Use the IntersectionObserver
API to load images dynamically.
Conclusion
Mastering these advanced JavaScript concepts enhances the efficiency and scalability of web applications. Whether enabling real-time communication with WebSockets, improving offline functionality with Service Workers, or leveraging WebAssembly for high-performance computing, these techniques equip developers with powerful tools to build modern web applications efficiently.
Which of these concepts are you most excited to implement? Let me know in the comments!
Leave a Comment